Javascript RegEx Tester
Javascript
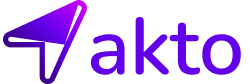
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction on Javascript Regex
JavaScript regular expressions are a powerful tool for pattern matching and text manipulation. Utilizing the built-in capabilities of JavaScript, developers can perform complex operations on strings, such as validation, parsing, searching, and replacing.
Core Constructs of JavaScript Regex
JavaScript regex operates with various constructs, each serving specific purposes:
Metacharacters
.
: Matches any single character, except newline characters.^
: Asserts the start of a line or string.$
: Asserts the end of a line or string.|
: Acts as a logical OR operator.
Character Classes
[abc]
: Matches any one of the charactersa
,b
, orc
.[^abc]
: Negates the set; matches any character excepta
,b
, orc
.[a-zA-Z]
: Specifies a range, matching any letter froma
toz
orA
toZ
.
Predefined Character Classes
\\d
: Matches any digit, equivalent to[0-9]
.\\D
: Matches any non-digit.\\s
: Matches any whitespace character.\\S
: Matches any non-whitespace character.\\w
: Matches any word character (alphanumeric & underscore).\\W
: Matches any non-word character.
Quantifiers
``: Zero or more times.
+
: One or more times.?
: Zero or one time; also used to denote a non-greedy quantifier.{n}
: Exactlyn
times.{n,}
:n
or more times.{n,m}
: Betweenn
andm
times, inclusive.
Special Constructs
(abc)
: A capturing group that matches the sequenceabc
.(?:abc)
: A non-capturing group.(?=abc)
: A positive lookahead, asserts that what follows isabc
.(?!abc)
: A negative lookahead, asserts that what follows is notabc
.
Anchors and Boundaries
\\b
: Matches a word boundary.\\B
: Matches a non-word boundary.\\A
: Matches the beginning of the input.\\Z
: Matches the end of the input, before a final newline (if any).
Flags
i
: Case-insensitive matching.g
: Global search.m
: Multi-line search.u
: Unicode; treat a pattern as a sequence of Unicode code points.y
: Sticky; matches only from the index indicated by thelastIndex
property of this regular expression in the target string.
JavaScript Regular Expressions Examples
Example 1: Email Validation
Example 2: Password Strength Check
Example 3: Extracting Words from a String
Practical Tips for JavaScript Regular Expressions
Familiarize yourself with the different flags and how they alter the behavior of your regex.
Use the
RegExp
constructor when you need dynamic patterns, but be mindful of the need to escape backslashes.Regular expressions in JavaScript are stateful when used with global or sticky flags. Be aware of the
lastIndex
property.Test and debug your regular expressions thoroughly. Tools like regex101 or Akto regex tester can be invaluable for this.
For complex patterns, consider breaking them down into smaller parts or using verbose mode (comments and whitespace in pattern) if using a transpiler or build tool that supports it.
Remember that JavaScript regex is capable of handling Unicode characters but requires the
u
flag for full Unicode support.Use non-capturing groups (
(?:...)
) when you don't need to save the captured match.Practice writing regular expressions for common tasks like email and URL validation, but also explore ready-made solutions for more reliable and maintainable code.
Be mindful of potential performance issues in large-scale applications, as complex regex can be computationally expensive.
Leverage modern JavaScript features like template literals for more readable and maintainable regex patterns.
These tips and examples should provide a solid foundation for working with regular expressions in JavaScript. Remember to utilize tools like Akto's regex tester for efficient debugging and validation of your regex patterns.