Secure Coding Practices
Secure coding is the practice of writing software to prevent vulnerabilities and security threats, reducing the risk of breaches, unauthorized access, and other issues.
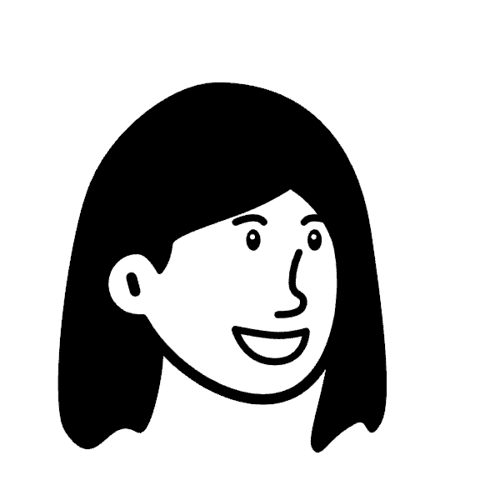
Insha
Oct 10, 2024
Secure coding is the practice of writing software code in a way that protects it from vulnerabilities and security threats. Developers apply specific techniques, such as input validation, encryption, and error handling, to ensure that the code is resistant to attacks. By following secure coding principles, developers reduce the risk of data breaches, unauthorized access, and other security issues, keeping applications safe and reliable.
In this blog, you will learn essential secure coding practices to protect the software from vulnerabilities and ensure reliability.
What is Secure Coding?
Secure coding involves writing software with a focus on preventing security threats and vulnerabilities from the start. Developers and security engineers actively identify and address potential risks, such as injection attacks
, buffer overflows, and insecure authentication
methods.
By prioritizing security during development, they reduce the likelihood of data breaches, financial losses, and system compromises. Insecure code allows attackers to exploit weaknesses, leading to reputational damage, loss of customer trust, and regulatory penalties.
Why is Secure Coding Important?
Secure coding is vital because it protects applications from exploitation, ensuring that developers and security engineers address vulnerabilities during the development process rather than after a breach occurs.
For instance, consider a login form that does not sanitize user input; this opens the door to SQL injection
, where attackers can bypass authentication and access sensitive user data. By applying secure coding principles such as input sanitization
and parameterized queries, developers and security engineers can close this vulnerability, preventing unauthorized access.
Beyond preventing specific attacks, secure coding practices help organizations avoid the financial and reputational fallout that often accompanies data breaches. It reduces the likelihood of costly security patches, legal penalties, and loss of customer trust.
Additionally, secure coding supports regulatory compliance (such as GDPR
or HIPAA), ensuring that applications meet the necessary security standards. In a practical sense, adopting secure coding from the start creates a more robust, reliable software environment that stands resilient against emerging cyber threats, ensuring that organizations can operate securely and effectively.
Common Security Vulnerabilities
Let's explore the most prevalent security vulnerabilities that threaten software applications today. Recognizing these risks empowers developers and security engineers to implement robust defenses and create more secure systems. Some common security issues in software include:
Injection Flaws
Attackers exploit injection flaws by inserting malicious code into a program, typically through unvalidated input fields. For instance, an attacker may enter malicious SQL commands like '; DROP TABLE users;--
into a login form.
If the application does not properly validate or sanitize the input, the database could execute the command, potentially deleting or corrupting critical data. Injection flaws, such as SQL injection
, are among the most dangerous vulnerabilities because they give attackers direct access to a system’s data or even allow them to take control of the application.
Cross-Site Scripting (XSS)
Hackers exploit cross-site scripting (XSS) vulnerabilities by injecting malicious scripts into web pages that other users view. For example, an attacker might input <script>alert('Hacked!');</script>
into a comment box on a website.
If the application doesn't properly sanitize this input, the script runs on the web page, causing the alert to display to anyone visiting that page. More dangerously, attackers could use XSS to steal cookies, session tokens, or other sensitive information from users, compromising their accounts or allowing unauthorized access to their data. Read more here.
Cross-Site Request Forgery (CSRF)
Attackers exploit CSRF vulnerabilities by tricking users into performing unintended actions on web applications without their knowledge. For example, an attacker might craft a malicious link that, when clicked, causes a logged-in user to unknowingly transfer money or change account settings. Since the user is authenticated
, the web application believes the request is legitimate, even though the user didn’t initiate the action. For more details, read the CSRF comprehensive guide.
Buffer Overflows
Buffer overflows happen when a program receives more data than expected, causing the excess to overwrite adjacent memory. This can result in crashes or allow attackers to execute malicious code. For example, if a program expects a username of 10 characters
but accepts 100 without validation
, the overflow can disrupt memory and alter system behavior. Attackers can exploit this to inject harmful code and take control of the system.
Insecure Direct Object References (IDOR)
Attackers exploit IDOR by manipulating object identifiers in URLs or parameters to access unauthorized data. For example, if a user changes the URL from example.com/user/123
to example.com/user/124
, they could access another user’s information if the system doesn’t properly validate permissions. This flaw occurs when the application fails to enforce proper access controls, allowing attackers to view, modify, or delete data that doesn't belong to them. Learn more about IDOR here.
Secure Coding Practices
Secure coding practices mean following specific rules to write safe and secure software. Some basic principles include:
1. Validate Input
Validating input ensures that user-provided data is safe and conforms to expected formats, preventing malicious inputs from exploiting the system.
By actively checking and sanitizing inputs, organizations can block common vulnerabilities like SQL Injection, where attackers attempt to manipulate database queries, and cross-site scripting (XSS), where malicious scripts are injected into web pages.
Input validation involves enforcing strict data types, lengths, and patterns, as well as escaping special characters to prevent harmful execution.
Example: Input Validation in Python
Let's examine a Python code snippet that demonstrates effective input validation:
The validate_input
function checks whether the input contains only alphanumeric characters. If the input is invalid, it raises a ValueError
. A try-except block then handles this error, allowing the program to inform the user about the invalid input without causing the program to crash. This approach ensures that the program manages errors smoothly and the system maintains user experience even when input validation fails.
2. Least Privilege
The principle of least privilege ensures that users and systems are granted only the minimum access required to perform their tasks. By limiting permissions, security engineers reduce the risk of unauthorized access to sensitive data or critical systems.
If a security breach occurs, attackers will have minimal access, limiting the potential damage they can cause. Regularly reviewing and updating access controls
helps ensure that no user or system has more privileges than necessary.
Example: Database Access in Python
Here's an example of implementing the principle of least privilege in Python when accessing a database:
The 'user': 'app_user'
connects to the database using a user account with limited privileges, such as read-only access to specific tables. When performing operations like SELECT * FROM sensitive_data
, the restricted user helps minimize the risk of damage in case of a security breach.
By using a database user with the least privileges necessary, organizations ensure that even if the application is compromised, attackers have limited access, reducing the potential impact on the system. This approach strengthens security by restricting permissions to only what is required.
3. Error Handling
Handling errors gracefully involves catching and managing errors without exposing sensitive technical details to potential attackers. When an error occurs, the system should display a generic message to the user while logging the specific issue internally for developers.
This prevents attackers from gaining insights into the system’s structure or vulnerabilities, reducing the risk of information disclosure
. Implementing proper error handling ensures that the application remains secure and user-friendly, even in the event of unexpected failures.
Example: Error Handling in Java
Let's examine a Java code snippet that demonstrates effective error handling:
The divide
method attempts to divide two integers. If division by zero occurs, it throws an ArithmeticException
. The try-catch block catches this exception and provides a user-friendly message, preventing the program from crashing and avoiding the exposure of technical details about the error. This approach ensures the application continues running smoothly while keeping sensitive information secure.
4. Code Reviews
Code reviews involve systematically examining source code to identify and fix security issues early. This process ensures code quality, consistency, and security by validating inputs, handling errors, and promoting best practices. Regular reviews enhance code readability, performance, and functionality
while also fostering collaboration and knowledge sharing among developers and security engineers.
Tools for Code Reviews
GitHub Pull Requests
GitLab Merge Requests
Bitbucket Code Reviews
SonarQube
Codacy
Checkmarx
5. Data Sanitization
When writing secure code, always sanitize user inputs to ensure only valid, safe data enters the organization’s system. Data sanitization removes unwanted or harmful content, preventing attacks like SQL injection, cross-site scripting (XSS), or buffer overflows. Security teams should validate input types, strip out dangerous characters, and use appropriate libraries or built-in functions for sanitization.
Data Sanitization Methods
Sanitizing data removes or alters any harmful parts to make it safe for use. Here are some methods:
Escaping: Security teams escape special characters by converting them to safe versions, like turning
<
into<
in HTML. This prevents attackers from injecting malicious scripts into the application. Escaping is particularly useful forHTML
,JSON
, and JavaScript outputs to stop XSS attacks.Encoding: Encoding changes data into a safer format for storage or transmission. For example, security engineers use URL encoding to ensure special characters in URLs are interpreted correctly or
Base64
encoding for sending binary data via APIs.Stripping: Stripping removes harmful characters or code entirely. For example, removing script tags from user input in a comment section prevents JavaScript from being executed. This approach is effective in reducing attack surfaces when users input large blocks of text.
6. Authentication and Authorization
To secure the application, enforce strong authentication methods by requiring users to create complex passwords and securely store them using hashing algorithms like bcrypt
or Argon2
. Always protect login data by using HTTPS to encrypt it during transmission over the internet. Implement multi-factor authentication
(MFA) to add an extra layer of security. Ensure that users only have access to the features and data they need by enforcing strict authorization controls.
Strong Passwords and Hashing
Implementing strong password policies and secure hashing techniques fortifies an organization's defense against unauthorized access and data breaches.
Example: Storing Passwords Securely in Python
In this code, the bcrypt
library in Python securely stores passwords. The hash_password
function first generates a unique salt with bcrypt.gensalt()
and then hashes the provided password with bcrypt.hashpw()
, combining the password and salt to produce a secure hash.
The check_password
function verifies if the input password matches the stored hashed password by comparing them with bcrypt.checkpw()
. This process ensures that even if passwords are compromised, the system securely hashes them and makes them difficult to reverse.
The use of salt adds an extra layer of security, ensuring that identical passwords produce different hashes, protecting against rainbow table attacks. When a user logs in, the system compares the provided password to the hashed value stored in the database, ensuring secure authentication.
Role-Based Access Control (RBAC)
RBAC helps manage what users can and cannot do within the application. Assign users to roles based on their responsibilities, and give each role specific permissions.
Example: Implementing RBAC in Python
In this code, a simple role-based access control (RBAC) system is implemented. The roles
dictionary defines different roles and their associated permissions. For example, the admin
role can view, edit, and delete data, while the user
role is limited to viewing data.
The check_permission
function checks if a specific role has a particular permission by looking it up in the roles
dictionary. It takes two arguments—role and permission—and returns True
if the role has the required permission, or False
if it does not.
In the usage example, the code checks if the admin
role has the edit_data
permission. Since the admin has this permission, the function prints a message confirming access. This method helps security teams manage and verify role-based permissions efficiently.
Final Thoughts
Secure coding is essential to protect the organization from threats and vulnerabilities. Always validate and sanitize inputs, use strong authentication, and manage sessions securely. Encrypt sensitive data, handle errors properly and keep the dependencies up-to-date. Regularly review and test the application code to maintain security. By following these practices, organizations can create reliable and secure software that users trust.
Akto's powerful platform enhances API security. Akto automates API security testing and provides real-time monitoring and automated checks to help organizations identify and mitigate risks effectively. Organizations can explore how Akto's solutions strengthen their APIs and strengthen their overall security posture by booking a demo.
Keep reading
News
5 mins
Akto Earns 20 Badges in G2’s Winter 2025 Reports for API Security and DAST
We’re thrilled to announce that Akto has been recognized as a High Performer in both API Security and Dynamic Application Security Testing (DAST) in G2’s Winter 2025 Reports.
API Security
8 Minutes
Top 10 Invicti Alternatives in 2025
In this blog, explore the top 10 Invicti Security alternatives and competitors, including key features and comparisons to help you choose the best solution.
API Security
3 minutes
What is API Discovery?
API Discovery helps identify, map, and manage APIs within an organization, ensuring security, performance, and seamless integration across systems.
Experience enterprise-grade API Security solution