PHP Injection
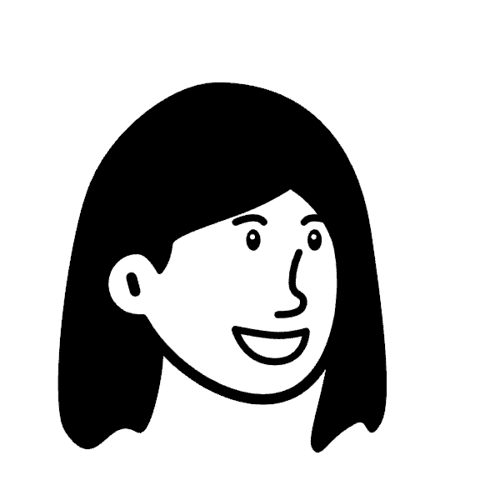
Insha
Nov 7, 2024
PHP injection is an attack where an attacker injects malicious PHP code into a vulnerable application, causing it to execute unintended commands. This can occur when unsanitized user inputs pass directly to PHP functions. The attacker can manipulate the application’s behavior, potentially leading to data theft, unauthorized access, or system compromise. Proper input validation and sanitization help prevent PHP injection attacks.
This blog explores PHP injection, including how it occurs, its impact, various vulnerable PHP functions, and secure coding practices.
What is PHP Injection?
PHP injection refers to a type of security vulnerability that allows attackers to inject malicious code into a PHP application, potentially leading to unauthorized access
or control over the server. This vulnerability primarily arises from improper handling of user input, allowing attackers to manipulate the execution of PHP scripts.
PHP Injection Example
Here's an example of PHP code vulnerable to PHP injection, with an explanation of the vulnerability:
In this scenario, the server retrieves user input from the URL query parameters using $_GET['user_input']
, a common method for obtaining data from forms or URLs. The server then uses the eval()
function to execute the user-supplied input as PHP code, treating it as part of the server’s code execution.
This practice introduces a critical vulnerability because it allows the server to execute any PHP code entered by the user without validation or sanitization. As a result, an attacker can exploit this weakness by passing malicious PHP code through the query parameter, leading to arbitrary code execution.
The impact of such a vulnerability can be severe, potentially allowing unauthorized access to sensitive information
, manipulation or deletion of data, or even full control of the server, depending on the attacker’s intent.
Impact of PHP Injection
PHP injection can have severe consequences, ranging from minor disruptions to complete system compromise, depending on the attacker’s intent and the level of access gained.
Remote Code Execution (RCE)
The most dangerous outcome of PHP injection is Remote Code Execution. Attackers can execute arbitrary PHP code on the server, gaining control over its functions and behavior. With RCE, attackers can manipulate the server to install malware, modify or delete files
, or create backdoors for future access.
Data Theft or Loss
PHP injection can expose sensitive data, including personal user information, database credentials
, or application settings
. Attackers can extract valuable data such as usernames, passwords, or financial details, leading to privacy breaches, identity theft, and reputational damage for the organization.
Privilege Escalation
Attackers can escalate privileges through PHP injection, gaining higher-level access
than originally intended. By executing unauthorized PHP code, an attacker may exploit system vulnerabilities to move from a limited user account to an administrative one, taking full control of the system.
Application Defacement
PHP injection allows attackers to modify server-side code
, which can lead to defacing the website or application. They may insert malicious content, redirect users to phishing sites, or display unwanted messages, damaging the organization’s brand and trust.
Denial of Service (DoS) Attacks
Attackers can also use PHP injection to create conditions for denial of service. They can flood the server with excessive commands, exhaust system resources
, or create infinite loops in the code, resulting in the application crashing or becoming unavailable.
Server Compromise
In the most extreme cases, PHP injection can lead to a complete server compromise. By gaining control of the web application’s PHP code, attackers may access other parts of the system, allowing them to launch additional attacks, pivot to other servers in the network, or gain persistent access to the infrastructure.
Common Vulnerable PHP Functions
Functions that execute PHP code dynamically or interact with user input in unsafe ways can cause PHP injection vulnerabilities. Here are some common functions that can result in these vulnerabilities:
eval()
The eval()
function poses a high risk for PHP injection as it evaluates a string
as PHP code. If developers pass user-supplied data to this function without proper validation, attackers can inject and execute arbitrary PHP code, leading to full control over the server's execution flow.
include() and require()
Using include()
or require()
functions to dynamically load PHP files based on user input introduces significant risks. Without validating the input, attackers can manipulate the file path to include malicious files, leading to code injection
and execution of unauthorized scripts.
preg_replace()
When development teams uses preg_replace()
with the deprecated 'e' modifier, it can execute PHP code contained in the replacement string. If user input is used to construct this string without proper validation, it opens the door for attackers to inject malicious code, leading to PHP injection.
exec() and system()
The exec()
and system()
functions execute shell commands on the server. If development teams passes user input directly to these functions without sanitization, attackers can inject arbitrary shell commands, compromising the system by executing unauthorized actions or gaining control of server resource.
mysqli_query() and PDO::query()
SQL injection vulnerabilities, which may escalate to PHP injection, can arise when mysqli_query()
or PDO::query()
are used to concatenate user input directly into SQL queries. Failing to use parameterized queries or proper sanitization leaves the application vulnerable to attacks that compromise both the database and PHP execution.
How Does PHP Injection Occur?
Several common scenarios can lead to PHP injection due to the improper handling of user-supplied data within PHP-based web applications:
Unsanitized User Input
PHP injection can occur when a web application fails to properly validate or sanitize user-supplied data
, such as form inputs, URL parameters, or cookies. When the PHP code uses this unsanitized input, it can execute malicious scripts. This lack of input validation creates an easy entry point for attackers to inject harmful PHP code
that can manipulate the server’s behavior.
Dynamic Code Execution
Dynamic code execution through functions like eval()
or include()
presents a high risk for PHP injection when the application uses user input to construct arguments without proper validation. If the application dynamically builds and executes code based on user input, attackers can inject harmful code that the server executes as part of its operations, leading to severe security risks.
Database Queries
While SQL Injection directly targets the database, improper handling of user input in certain situations can also allow attackers to execute PHP code through the misuse of functions like eval()
, exec()
, or even through dynamically generated queries. In such cases, attackers can achieve similar outcomes to PHP injection, including remote code execution or data compromise.
File Inclusion
File Inclusion vulnerabilities occur when user input is used to include files in a PHP application without proper validation or sanitization. This can lead to PHP injection if attackers manipulate the file paths to include malicious PHP code or sensitive server files
.
In Local File Inclusion (LFI)
, the attacker exploits the vulnerability to include files from the local server, often leading to the exposure of critical files such as server configurations
or password files. If an attacker can also upload a file to the server, they may inject malicious PHP code and force the server to execute it, gaining unauthorized access.
Remote File Inclusion (RFI)
Remote File Inclusion (RFI) presents a more severe vulnerability that enables attackers to include files from remote servers via user-supplied input
. When developers improperly handle RFI, attackers can inject malicious PHP code hosted on an external server and execute it within the target application. This can result in full system compromise or even Remote Code Execution
(RCE).
In an RFI attack, an attacker typically passes a URL to the vulnerable PHP application, causing it to include and execute malicious scripts hosted remotely.
Secure Code Practices
PHP injection vulnerabilities require a multi-layered approach involving secure coding practices, input validation, sanitization techniques, and proper configuration of server-side components. These include:
Input Validation
Implement strict input validation to ensure that user-supplied data conforms to expected formats, data types, and length limits. By rejecting or sanitizing inputs that don't meet these criteria, the application can prevent attackers from injecting harmful code. Robust input validation is one of the most effective ways to mitigate PHP injection risks
.
Parameterized Queries
Using parameterized queries with PDO or mysqli when interacting with databases helps prevent SQL injection
and mitigates PHP injection. Parameterized queries separate the data from the executable code, ensuring that the application treats user input purely as data, not part of the SQL command or PHP logic.
Output Encoding
Encoding output data with functions like htmlspecialchars()
or htmlentities()
ensures that the application treats user input displayed in HTML as data, not code. This prevents cross-site scripting (XSS) attacks and reduces the risk of PHP injection. Proper encoding ensures that the application safely outputs any user input that contains special characters without executing it.
Whitelisting
Whitelisting acceptable characters or patterns during user input validation ensures that the application processes only safe input. Rejecting inputs that contain disallowed characters helps to prevent injection attacks, including PHP injection. This proactive approach limits attackers' ability to inject harmful code through unexpected or malformed inputs.
Content Security Policy (CSP)
Implementing Content Security Policy (CSP) headers restricts the sources from which the browser can load content, such as scripts. This helps mitigate the impact of PHP injection by preventing injected scripts from executing, offering a layer of defense against script-based vulnerabilities
like XSS.
Input Filtering
Using PHP filters like filter_input()
and filter_var()
helps validate and sanitize user input based on predefined filter types. Filters such as FILTER_SANITIZE_STRING
or FILTER_SANITIZE_EMAIL
ensure that inputs conform to expected data formats, reducing the risk of PHP injection by removing harmful elements before processing.
Web Application Firewall (WAF)
Deploying a Web Application Firewall (WAF) adds an extra layer of protection by filtering and monitoring incoming web traffic. A WAF can detect and block malicious requests that attempt to exploit PHP injection vulnerabilities, protecting the application from known attack patterns and threats.
Final Thoughts
PHP injection is a significant security risk that can allow attackers to run malicious code within applications. To protect against these vulnerabilities, implementing strong input validation and avoiding dynamic code execution is crucial.
Akto provides multiple pre-built injection test templates that help security teams identify and mitigate vulnerabilities like SQL injection, SSTI, and more. Application security engineers can explore the comprehensive injection test templates, including SSTI in Flask and Jinja, to strengthen the application's defenses. Check them out here and secure the application today!
Book the Akto Demo today!
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.