White Box Fuzzing
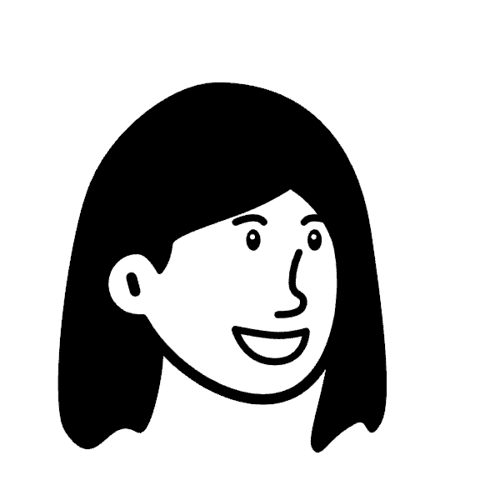
Insha
Nov 5, 2024
White Box Fuzzing is a software testing technique that involves generating inputs for an application by analyzing its source code. Unlike Black Box Fuzzing, it uses internal knowledge of the code to create targeted test cases, aiming to explore deeper code paths and uncover hidden vulnerabilities. By using code instrumentation, White Box Fuzzing increases the chances of detecting complex issues like buffer overflows and logic flaws.
This blog explores White Box Fuzzing, its benefits, how it works, a practical example, key techniques used in the process, and challenges of White Box Fuzzing.
What is White Box Fuzzing?
White Box Fuzzing tests a program by sending invalid or unexpected
inputs while using knowledge of its internal workings, such as source code and data structures
. This approach allows security teams and testers to create more targeted and effective test cases.
By leveraging access to the program's logic, White Box Fuzzing can uncover deeper vulnerabilities. Security teams and testers may also use model-based testing techniques
to generate inputs based on the program's specifications. The method provides a thorough and detailed assessment of the program's security.
Benefits of White Box Fuzzing
White box fuzzing offers several key benefits that enhance software security and improve the overall quality of applications.
Early Vulnerability Detection
White box fuzzing allows for early detection of vulnerabilities by thoroughly testing internal logic
and data flows
. It identifies potential security issues before attackers can exploit them, giving developers and application security engineers a head start in addressing flaws. This proactive approach strengthens the security posture of software, reducing the risk of late-stage vulnerabilities
.
Precise Test Case Generation
By leveraging access to source code and internal structures, white box fuzzing generates highly targeted test cases. These test cases aim at specific code paths
and functions, increasing the likelihood of uncovering critical vulnerabilities. This precision ensures that developers identify and address even subtle flaws within complex areas of the codebase.
Improved Code Coverage
White box fuzzing enhances code coverage by systematically exploring various code paths and branches. Techniques such as code coverage-guided fuzzing
enable comprehensive testing, ensuring that security teams and testers examine a broad range of program behaviors. This thorough approach results in more resilient software by detecting vulnerabilities across multiple execution paths.
Enhanced Debugging Capabilities
White box fuzzing provides detailed insights into vulnerabilities by analyzing the program’s internal state and execution flow. This enables developers and application security engineers to pinpoint the root causes of issues quickly and efficiently. Detailed feedback reduces debugging time
and helps fix vulnerabilities with greater accuracy, streamlining the overall resolution process.
Integration with Development Workflows
White box fuzzing integrates seamlessly with existing development workflows, making it possible to continuously test and validate code changes. Automated fuzzing tools ensure that developers and application security engineers detect and address vulnerabilities early in the software development lifecycle
. This continuous integration helps maintain a high standard of security and software quality throughout the development process.
How White Box Fuzzing Works
White Box Fuzzing employs a systematic approach to test software applications by analyzing their internal structure and generating targeted inputs.
Creating the Input Model
White Box Fuzzing starts by building a model that defines the program's input format, outlining the structure and constraints of the data. This model specifies details like the length, type, and range of each input field. The fuzzer then generates numerous random inputs
that align with this model, ensuring that they mimic realistic data the program might encounter. This approach allows for more controlled and effective fuzz testing.
Generating and Feeding Inputs
Once the input model is set, the fuzzer creates and injects large volumes of inputs into the program. These inputs are designed to test the program's response to a variety of scenarios, from simple data entries to complex, malformed
inputs. The fuzzer closely monitors the program's behavior under these conditions to identify any issues that arise, such as crashes or unexpected errors
Detecting Anomalies
As the program processes each input, the fuzzer looks for abnormal behavior
, including memory leaks, buffer overflows
, and division by zero errors. When the fuzzer detects an anomaly, it logs the specific input that triggered
it. This anomaly becomes part of a set of test cases, helping focus further investigation on inputs that reveal potential vulnerabilities.
Analyzing Program Behavior
The fuzzer uses advanced techniques like symbolic execution
and taint analysis
to perform deeper analysis around detected anomalies. These methods trace the path of the error, identifying the specific conditions
that led to the vulnerability. Based on this information, the fuzzer can generate new inputs that target and exploit the detected weaknesses more effectively.
Validating and Prioritizing Vulnerabilities
After identifying potential vulnerabilities, the fuzzer uses automated tools and techniques to validate them. This may involve static analysis, dynamic analysis
, or manual code review to confirm the findings. The fuzzer then prioritizes the vulnerabilities based on their severity, providing a list of confirmed security issues that developers and application security engineers can address in order of importance.
Techniques Used in White Box Fuzzing
White box fuzzing employs various techniques to thoroughly test software applications and uncover potential vulnerabilities. These techniques leverage internal knowledge of the program to create targeted and effective test cases.
Code Coverage Guided Fuzzing
Code Coverage Guided Fuzzing monitors which parts of the code are executed when test inputs are run. By tracking code coverage, the fuzzer can generate inputs that target unexplored sections of the program, increasing the chances of finding vulnerabilities.
This technique systematically drives the testing process toward untested paths, ensuring broad coverage of the application's functionality. For instance, tools like AFL (American Fuzzy Lop
) use this approach to guide the fuzzing process.
Mutation-Based Fuzzing
Mutation-Based Fuzzing generates new test cases by modifying existing inputs. Mutations can include bit flips, byte insertions
, deletions, or other random changes to the input data. This approach explores different behaviors in the program by altering input values and observing how the application responds. By randomly applying mutations
, the fuzzer can uncover unexpected issues in the program's input handling.
Grammar-Based Fuzzing
Grammar-Based Fuzzing generates test cases by following a defined grammar or syntax of the input format. This technique ensures that the generated inputs are valid and conform to the expected structure
of the application’s input, increasing the likelihood of exploring relevant code paths. By focusing on the correct input format, grammar-based fuzzing can expose vulnerabilities tied to specific input structures.
Feedback-Driven Fuzzing
Feedback-Driven Fuzzing uses program behavior, such as crashes, assertions
, or other indicators of abnormal
execution, to guide the generation of new inputs. By analyzing the feedback from the application’s responses, the fuzzer adjusts its approach to focus on inputs that are more likely to reveal vulnerabilities. This dynamic adjustment makes feedback-driven fuzzing highly effective in finding deeper and more critical issues within the program.
Symbolic Execution
Symbolic Execution is a powerful technique that executes the program with symbolic inputs instead of concrete values. These symbolic inputs
represent multiple possible values, allowing the fuzzer to explore different paths within the program simultaneously. By using symbolic execution, the fuzzer can systematically examine all possible execution paths and identify vulnerabilities related to specific input conditions.
Concolic Testing
Concolic Testing combines concrete and symbolic execution to systematically explore program paths and increase code coverage. By using concrete inputs
to guide the execution and symbolic inputs to explore alternative paths, concolic testing achieves deeper program analysis. This hybrid approach allows the fuzzer to discover vulnerabilities that traditional fuzzing techniques may not expose.
Practical Example: White Box Fuzzing with KLEE
KLEE
, a symbolic execution tool that automatically generates test cases for programs. KLEE particularly focuses on discovering vulnerabilities by exploring all possible code paths.
KLEE analyzes the source code at a granular level
and generates inputs that systematically trigger execution paths based on constraints identified during the analysis. This approach gives KLEE a significant advantage in thorough program testing.
Step 1: Install KLEE and Compile the Target Program
To fuzz a simple program with KLEE, Security teams first need to compile the target program using LLVM:
Step 2: Run KLEE on the Program
Once security teams compile the program, KLEE can symbolically execute it, exploring different paths based on the conditions found in the code:
This command runs KLEE on the LLVM bitcode file (program.bc
). KLEE will analyze all possible execution paths and generate test cases that trigger bugs or edge cases in the program's logic.
Step 3: Analyze the Results
KLEE generates test cases that expose vulnerabilities such as segmentation faults
, buffer overflows, or other memory-related issues. The output includes files containing inputs that lead to different execution paths.
For instance, KLEE might discover that a certain input value causes a division by zero, which the security teams can then investigate and resolve.
Example Program in C
In this program, KLEE makes the variable y
symbolic, which means that instead of assigning it a fixed value, KLEE will explore all possible values for y
during symbolic execution. It will discover that when y = 0
, the program executes the error message due to division by zero, identifying a potential issue.
Step 4: Review the KLEE Output
KLEE generates multiple test cases, one of which will show that when y = 0
, the program prints an error. This test case highlights a potential vulnerability in how the program handles user input.
Security teams can inspect the test cases generated in the klee-out-*
directories:
This command provides detailed statistics
about the test cases generated, including how many unique execution paths security teams explored and how many tests led to uncovered vulnerabilities.
Challenges of White Box Fuzzing
White box fuzzing is a sophisticated testing technique that leverages knowledge of a program's internal structure to identify vulnerabilities. Despite its benefits, this approach faces several significant challenges:
1. Path Explosion
Path explosion poses one of the most critical challenges in white box fuzzing. As program complexity increases, the number of execution paths grows exponentially. This especially challenges programs like parsers or interpreters
that handle various input formats and branching logic.
The exponential growth of possible execution paths can overwhelm the fuzzer, causing it to explore trivial or redundant paths
instead of focusing on those more likely to expose critical vulnerabilities. Developers and application security engineers can apply techniques like constraint pruning
or heuristics
to mitigate this issue, but these approaches may miss some deeper vulnerabilities.
2. Symbolic Execution Limitations
White box fuzzing relies heavily on symbolic execution
, which treats program variables as symbolic rather than concrete values. This allows the fuzzer to explore multiple paths in a single run. However, symbolic execution encounters performance bottlenecks, particularly when programs involve long traces
or generate numerous constraints.
As program logic becomes more complex, especially when involving pointers or external libraries, the symbolic execution engine struggles to keep up. This limitation often results in incomplete coverage
of potential vulnerabilities, forcing fuzzers to compromise between speed and depth of analysis.
3. Constraint Solving Challenges
The performance of constraint solvers closely ties to the effectiveness of symbolic execution in white box fuzzing. These solvers must quickly handle numerous conditions arising from different code paths, but solving these constraints can slow down, especially in complex programs.
As the number of conditions increases, so does the difficulty in finding solutions within a reasonable time frame. While advanced solvers can manage many constraints, they are not perfect. Long delays in constraint solving can significantly reduce the fuzzing process's efficiency. Techniques like caching
frequently solved constraints can help but do not fully resolve the issue.
4. Input Model Complexity
Generating an accurate input model for white box fuzzing presents a significant challenge, especially for programs handling complex, structured data formats. Real-world applications often involve inputs like XML
, JSON, or custom file formats, which require detailed modeling to generate meaningful and valid test cases.
An inaccurate or incomplete input model may cause ineffective fuzzing that fails to explore the program's critical areas, missing important vulnerabilities. Grammar-based fuzzers can mitigate this issue by providing structured input generation, but building such grammars demands significant time and effort.
5. Resource Intensity
White box fuzzing consumes substantial computational power
, memory, and time to execute effectively. Symbolic execution and constraint solving, key components of this approach, can deplete a lot of system resources.
This resource intensity limits white box fuzzing's application in environments with constrained resources or scenarios requiring rapid testing. Cloud-based or distributed fuzzing can alleviate some of these resource constraints by spreading the workload across multiple machines, but the high cost of resources remains a limiting factor for many organizations.
Final Thoughts
White box fuzzing proves to be an integral part of the cybersecurity landscape. It provides organizations with a proactive and detailed approach to uncovering potential vulnerabilities in software. By leveraging code access and internal structures, it allows for precise test case generation, improved code coverage, and early vulnerability detection. Furthermore, it enhances debugging capabilities and integrates seamlessly into development workflows - all contributing to effective risk mitigation.
Akto, an API security platform, offers powerful capabilities for performing API fuzzing. It can automatically test the APIs for various vulnerabilities, helping application security engineers catch security flaws and performance issues early. With Akto, security engineers can integrate fuzz testing seamlessly into the API security workflows. To see how Akto can help secure the APIs, book a demo today!
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.