Restler - REST API fuzzer
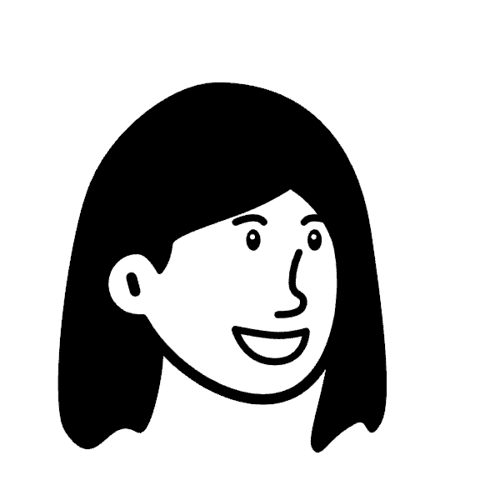
RESTler is a fuzzing tool designed for testing REST APIs by generating requests based on the API’s specifications. It uses machine learning to explore different input combinations, detecting vulnerabilities such as crashes and security flaws. RESTler works by automatically creating sequences of API requests, focusing on areas of the API that may be less tested.
This blog explores RESTler: what it is, why to use it, how it works, a step-by-step guide for its usage, and best practices for effective implementation.
What is RESTler?
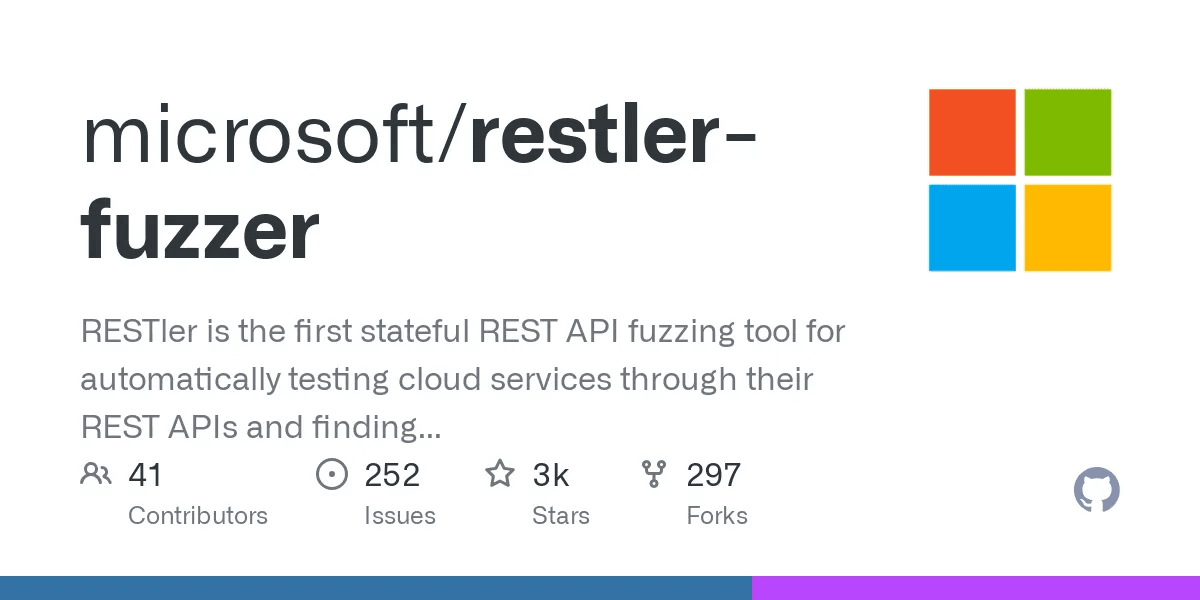
Microsoft developed RESTler as an automated tool to test RESTful web services by leveraging fuzzing techniques and dynamic analysis. It generates numerous HTTP requests with a variety of inputs and monitors the responses to detect
bugs, vulnerabilities, and security issues in REST APIs.
Unlike traditional fuzzers, RESTler understands the dependencies between API endpoints, enabling it to create sequences of requests that test more complex API behaviors. By exploring different combinations of inputs and request sequences, RESTler
helps uncover issues such as crashes, security flaws, and unexpected behaviors in RESTful APIs. This makes it an effective tool for improving the robustness and security of modern web services.
Why use RESTler?
RESTler offers several compelling reasons for its adoption in API testing and security analysis, empowering developers and security engineers with powerful capabilities. These include:
Automation
RESTler automates the process of testing RESTful APIs by generating a large number of test cases
with varying inputs. This eliminates the need for manual testing and allows for more comprehensive and efficient coverage of potential vulnerabilities
. The automation feature ensures that even complex APIs are tested systematically.
Coverage
RESTler explores a wide range of input values, request parameters
, and API endpoints, which helps uncover bugs and vulnerabilities that traditional testing methods may overlook. Its deep exploration of API interactions ensures that all areas of the API, including edge cases, are thoroughly tested.
Speed
RESTler can execute thousands of test cases in a short period, allowing for rapid identification of issues. This speed enables security teams to quickly detect
and fix vulnerabilities or bugs before they affect users, enhancing the overall reliability and security of the API.
Scalability
RESTler scales efficiently to test APIs of varying sizes and complexities, from small microservices
to large distributed systems with multiple endpoints. Its ability to handle complex APIs with numerous dependencies makes it suitable for both small-scale and enterprise-level applications.
Regression Testing
RESTler is highly effective for regression testing, ensuring that changes or updates to the API do not introduce new bugs
or break existing functionality. By running automated tests against modified API endpoints, RESTler helps maintain the stability of the API over time.
Security Testing
RESTler uncovers security vulnerabilities such as injection flaws, authentication bypasses, and data exposure issues within APIs. By identifying these vulnerabilities, RESTler contributes to strengthening the security posture of the application, protecting it from potential attacks.
How Does RESTler Work?
RESTler operates by leveraging fuzzing techniques to test RESTful APIs in a structured and automated manner. Unlike traditional fuzzers, which might send random inputs to endpoints, RESTler takes a more intelligent approach by using the API’s specification (like OpenAPI
or Swagger files
) to generate meaningful and ordered requests. Here's how RESTler works:
1. API Specification Parsing
RESTler begins by parsing the API’s specification document (OpenAPI/Swagger), which provides details about the API’s endpoints, parameters, and expected behaviors
. This specification is essential because it informs RESTler about the API’s structure and allows RESTler to generate valid requests.
2. Grammar Generation
Once RESTler has parsed the API specification, it compiles the information into a grammar file
. RESTler creates this grammar file as a set of rules that describe how different API requests should be structured, including the parameters, methods, and endpoints. RESTler generates this grammar to reflect how requests should be formed and how various inputs can be combined for thorough testing.
3. Stateful Testing and Sequence Generation
Unlike simple fuzzers that send one-off, stateless requests, RESTler performs stateful testing
. It understands the dependencies between API endpoints, meaning some endpoints need to be called in a certain sequence to change the system's state.
For example, an "order" endpoint may require an "authentication" or "create user" request to be successfully called first. RESTler intelligently creates sequences of API calls to simulate real-world usage patterns
, allowing it to find bugs related to complex interactions between API endpoints.
4. Fuzzing Requests
After generating the grammar and request sequences, RESTler begins fuzzing. It sends numerous API requests with different combinations of inputs, both valid and invalid, to test the API’s response. RESTler’s fuzzing capabilities include testing for:
Malformed Requests: RESTler generates inputs that intentionally violate the expected structure of the API. This includes omitting required parameters, providing
incorrect data types
, or sending malformedJSON payloads
. By doing so, it tests the API's robustness in handling invalid inputs and its ability to provide meaningful error messages.Edge Cases: The tool explores unusual or boundary input values that might not be covered in typical testing scenarios. This includes testing with
extremely large or small numbers
, empty strings, orspecial characters
. By pushing the limits of acceptable inputs, RESTler aims to uncover potential vulnerabilities or unexpected behaviors in the system's data handling.Unexpected Inputs: RESTler goes beyond typical use cases by sending data that falls outside the expected range or format. This could involve injecting
SQL queries
, cross-site scripting (XSS) payloads, or other potentially malicious inputs. The goal is to identify any weaknesses in input validation or sanitization that could lead to security vulnerabilities, crashes, or unintended system behaviors.
5. Dynamic Analysis and Real-time Feedback
As RESTler fuzzes the API, it dynamically monitors the responses from the API in real-time. It looks for anomalies such as error codes
, crashes, and other unexpected behaviors
. Based on this feedback, RESTler adjusts its strategy to focus on areas of the API where issues have already been detected. This feedback-driven fuzzing
ensures that RESTler’s testing is both efficient and targeted, uncovering deeper bugs as it continues testing.
6. Bug Detection and Replay
Once RESTler has completed its fuzzing process, it records all the bugs and anomalies it identified, such as server crashes, security flaws
, or unexpected behavior. RESTler stores these results in detailed logs. These logs can be used to replay the bug-inducing requests
, which helps developers and application security engineers reproduce the issues and fix them more easily. This replay feature is crucial for debugging as it enables developers and security engineers to analyze the specific conditions that caused a failure.
7. Regression Testing
RESTler is also effective for regression testing
. Once an API has been updated or patched, RESTler can rerun its tests to ensure that new changes haven't introduced additional bugs or broken existing functionality. This makes RESTler not only a tool for initial security testing but also a valuable asset in maintaining the long-term stability
and security of APIs.
Step By Step Guide on Using RESTler
Follow this comprehensive guide to set up and utilize RESTler effectively for API fuzzing and security testing.
Set up RESTler
Before using RESTler, security teams should make sure that they have Python 3.8.2
or higher and the .NET Core SDK version 3.1
installed. Clone the RESTler Fuzzer repository from GitHub and compile it by running the following commands in the RESTler repository folder:
This command compiles RESTler and stores the output binaries in the specified restler_bin
directory, making it ready for fuzzing tasks.
Compile API's Grammar
RESTler uses the API’s specification (OpenAPI or Swagger) to create a grammar file, which will be used to generate test cases.
First, download the API’s OpenAPI or Swagger specification file (typically a
.json
file that describes the API’s structure and endpoints).Once you have the API specification file, run the following command to compile the API’s grammar:
Replace <full_path_to_openapi.json>
with the full path to the downloaded API specification file. RESTler will parse the specification and generate a grammar file that describes how to send requests to the API.
Test the Grammar
After compiling the API’s grammar, it’s important to test that RESTler can correctly interpret the grammar and interact with the API. Use the following command to run a test:
<full_path_to_grammar.py>
: The path to the grammar file generated in the previous step.<full_path_to_dict.json>
: The dictionary file used for fuzzing. RESTler generates this based on the API specification.<full_path_to_engine_settings.json>
: Settings for the RESTler engine. RESTler provides default settings, but security teams can customize them as needed.-no_ssl
: Use this flag if the API does not require SSL. If SSL is needed, omit this flag. This command ensures that RESTler can successfully parse the API’s specification and interact with the API’s endpoints. It provides coverage statistics that indicate how well the test run covers the API’s endpoints.
Fuzzing
Once the grammar is tested, RESTler can perform fuzzing to uncover security vulnerabilities and bugs. RESTler provides two modes for fuzzing: fuzz-lean
and fuzz
.
Fuzz-lean Mode: Executes one test per endpoint and method.
Fuzz Mode: This mode performs a more exhaustive fuzzing process by searching for bugs across the API using a breadth-first approach.
<full_path_to_grammar.py>
,<full_path_to_dict.json>
, and<full_path_to_engine_settings.json>
should be correctly specified as generated in previous steps.The
-time_budget
parameter specifies how long RESTler should run the fuzzing process. Set it to a value in hours based on how long you want to fuzz (e.g.,-time_budget 2
for two hours).
Debugging Bugs
Once RESTler completes the fuzzing process, it saves the results in the Fuzz/RestlerResults
directory, where security teams can find logs of any bugs discovered.
To replay the requests that triggered bugs, use the following command:
<path_to_the_log>
: This should point to the log file that contains the requests which led to a bug. This command replays the bug-inducing requests so that developers can analyze and fix the issue. It helps pinpoint exactly what caused the API to fail or behave unexpectedly.
Best Practices for using RESTler
To maximize the effectiveness of RESTler and ensure comprehensive API testing, consider implementing these best practices:
Utilize OpenAPI Specifications
RESTler generates fuzzing grammars from OpenAPI (or Swagger) specifications, which define the API’s structure, endpoints, and expected behavior. To maximize RESTler’s effectiveness, ensure that the API is well-documented
with a complete and accurate OpenAPI specification.
An incomplete or inaccurate specification will limit RESTler’s ability to explore all endpoints and detect vulnerabilities. By providing comprehensive documentation, security teams allow RESTler to generate meaningful and stateful sequences of requests that cover more API functionality.
Focus on CRUD Operations
RESTler works best with APIs that implement the basic CRUD
(Create, Read, Update, Delete) operations. These operations allow RESTler to create dependencies between API requests, which is crucial for stateful fuzzing.
For example, RESTler will issue a create
request before attempting to update
or delete
the resource, simulating real-world use cases. While RESTler can test APIs that don’t fully adhere to CRUD principles, it performs optimally when it can build request sequences that reflect how users actually interact with the API. Ensuring the API supports these core operations enables RESTler to uncover deeper bugs related to complex interactions.
Manage Dependencies Effectively
RESTler handles dependencies between API requests by ensuring that prerequisites, such as resource creation, are satisfied before other requests are issued. It’s important that the API is designed to handle the creation and deletion of resources smoothly so that RESTler can generate valid request sequences
.
For example, RESTler might need to create a user before testing an endpoint that modifies user information. If security teams do not manage dependencies well, RESTler may encounter issues in state management
, limiting its effectiveness. Ensuring the API’s endpoints are designed with clear dependencies helps RESTler maintain state across multiple requests, leading to more thorough fuzzing.
Implement Security Checkers
RESTler includes a variety of built-in security checkers
that test for common vulnerabilities, such as unauthorized access, SQL injection, or improper input validation. To fully leverage RESTler’s security capabilities, ensure that the appropriate security checkers are enabled during fuzzing.
Additionally, RESTler allows the customization of security checkers to address specific risks unique to the API. For instance, application security engineers can create checkers to test for custom authorization schemes or unique business logic flaws. Utilizing RESTler’s default and custom security checkers helps security teams proactively identify security weaknesses, thereby strengthening the API’s security posture and protecting against potential threats.
Monitor Resource Usage
Since RESTler generates a high volume of requests during fuzzing, it can lead to significant resource consumption on the API server. Without proper monitoring, this can result in resource exhaustion
, performance degradation, or even crashes
.
To mitigate these risks, implement monitoring tools like Prometheus
or Grafana
to track CPU, memory, and network usage while RESTler is running. Set up alerts if any resource usage thresholds are exceeded.
Final Thoughts
RESTler serves as a powerful tool for enhancing the quality of RESTful services. Its automated testing capabilities, wide coverage, and real-time feedback make it a valuable asset for any development team. It not only accelerates the process of identifying and resolving issues but also contributes significantly to improving the security of applications. However, It's crucial for security engineers to keep themselves updated with its features and usage to maximize its benefits fully.
Akto, an API security platform, offers powerful capabilities for performing API fuzzing. It can automatically test the APIs for various vulnerabilities, helping application security engineers catch security flaws and performance issues early. With Akto, application security engineers can integrate REST API fuzz testing seamlessly into the API security workflows. To see how Akto can help secure the APIs, book a Akto demo today!
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.