What is API Fuzzing?
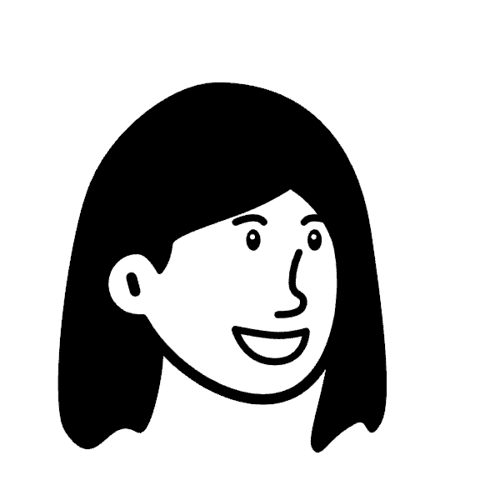
Insha
Nov 5, 2024
API fuzzing sends a wide range of unexpected or malformed inputs to an API to discover vulnerabilities or flaws. Testers use fuzzing tools to craft these inputs and observe the API's response to unusual or invalid data. This process aims to identify crashes, security vulnerabilities, or incorrect behaviors that standard testing might not reveal. By exposing APIs to unpredictable conditions, API fuzzing helps ensure their robustness and security.
This blog delves into API fuzzing, highlighting its significance in identifying vulnerabilities and ensuring robust API performance. It outlines various fuzzing techniques and tools that aid in uncovering security flaws.
What is API Fuzzing?
API fuzzing tests an application's API (Application Programming Interface) security and robustness by sending a large number of invalid, unexpected, or random inputs. This technique bombards the API with various data types to identify weaknesses or vulnerabilities. As a result, developers can uncover potential bugs, security loopholes, or unexpected behaviors in the API, enabling them to address these issues before they become problematic in production.
Why is API Fuzzing Important?
API fuzzing provides several key advantages that make it essential for API security and development.
Identifying Vulnerabilities
API fuzzing uncovers vulnerabilities by sending unexpected inputs that expose flaws like buffer overflows
, injection attacks, or improper error handling. Attackers can exploit these vulnerabilities to compromise the system’s security. By identifying these issues early, fuzzing strengthens the API's defenses against malicious attacks.
Testing Boundary Conditions
APIs often limit the inputs they can accept
, and fuzzing tests those boundaries. By sending inputs that push or exceed these limits, fuzzing ensures the API behaves correctly under extreme
conditions. This testing reveals issues that might not surface during regular testing.
Discovering Unexpected Behavior
API fuzzing exposes how an API handles invalid
or malformed inputs, often revealing unexpected
behaviors. These behaviors can indicate underlying security risks or implementation flaws. Identifying such issues early allows developers to fix them before exploitation.
Improving Test Coverage
Traditional testing might miss certain edge cases or input combinations, but fuzzing explores a much broader range of inputs. This approach increases test coverage and helps identify hidden bugs
that standard testing methods might overlook. Fuzzing ensures that the API undergoes testing against a wide variety of inputs.
Assessing Security Posture
API fuzzing provides a clear picture of your application's security posture by identifying weaknesses in its implementation. Fixing these vulnerabilities early enhances the API and reduces the risk of security breaches. Regular fuzzing helps maintain a strong security stance over time.
Compliance Requirements
Many industries mandate comprehensive security testing, making fuzz testing a crucial part of compliance standards. API fuzzing helps organizations meet these requirements and demonstrates their commitment to security best practices. It ensures that APIs align with industry regulations
and undergo thorough testing for potential vulnerabilities.
Types of API Fuzzing
API fuzzing encompasses several distinct techniques, each targeting different aspects of an API to uncover vulnerabilities and ensure robust performance.
1. Endpoint Fuzzing
Endpoint fuzzing tests API endpoints by sending unexpected or random data to observe their reactions. This technique resembles testing building entrances by trying various keys to find the one that unlocks the door.
For example, endpoint fuzzing involves sending different types of data to the API's endpoints to identify weaknesses or vulnerabilities in the API's security.
2. Input Fuzzing
Input fuzzing tests a program or system by providing unexpected or unusual data to observe its response. Security engineers deliberately send strange inputs to determine if the program can handle them without crashing or generating errors.
Scenario #1
An example of an HTTP request to an API endpoint with JSON
parameters is as follows:
In this example, the endpoint is /api/search
, and it utilizes a POST request. The content type is application/json
, indicating that the request body is formatted in JSON. The JSON object includes a search query and a limit parameter. The limit parameter contains an abnormally large value ("999999999999999999999"
), which could potentially overflow or cause unexpected behavior on the server. Fuzzing the JSON parameters with unexpected or malicious inputs tests how well the API handles such data and whether it validates and sanitizes inputs properly to prevent security vulnerabilities.
Scenario #2
Parameter fuzzing of an HTTP request involves altering the ID parameter
to observe how the server responds to different values:
In this request, the endpoint is /api/user
, with the ID parameter set to "123"
. By adjusting the ID to values such as "999"
, "admin"
, or special characters like "@#$%"
, security engineers can observe the server's reactions.
If the server fails to validate the input properly, it may expose vulnerabilities, such as Insecure Direct Object References (IDOR), allowing unauthorized users to access sensitive data simply by changing ID values. Such vulnerabilities can lead to significant security risks. Read more about IDOR here.
3. HTTP Method Fuzzing
HTTP Method Fuzzing tests a web server or application by sending different HTTP methods to an endpoint to observe its reactions. This process helps uncover unexpected behavior or vulnerabilities when the server handles various HTTP methods.
In this example, the request uses the POST method to send data to the /api/feedback
endpoint. The request body contains a JSON payload with the parameters user_id
and feedback
. By fuzzing the HTTP method, such as changing POST to GET, security engineers may trigger different functionalities. For instance, altering the method might reveal security flaws, like Broken Functionality Level Authorization (BFLA
), where different HTTP methods expose unintended behavior.
How to Perform API Fuzzing?
To perform API fuzzing effectively, follow these key steps to systematically test and secure your API.
1. Understand API Endpoints
Thoroughly understand the API you want to test. Review the documentation to learn about the API endpoints, request methods
, parameters, and expected responses. This information helps identify critical areas to target during fuzzing. Without a solid understanding, security engineers may miss important attack vectors or misinterpret test results.
2. Select Fuzzing Tools
Choose the appropriate tool for API fuzzing. Tools like Burp Suite, Postman, OWASP
ZAP, or AFL
(American Fuzzy Lop) offer robust fuzzing capabilities. Select a tool that aligns with testing goals, whether focused on security, performance, or general input validation. Evaluate tools based on their features, flexibility, and ease of integration with the testing environment.
3. Craft Fuzzing Payloads
Generate a variety of fuzzing payloads to test the API’s resilience against different inputs. These payloads can range from valid to malformed or unexpected data. By feeding unexpected inputs into the API, security engineers can trigger unhandled exceptions or logical
flaws. Tools like SecLists provide a wide range of pre-built payloads that target common vulnerabilities.
4. Define Fuzzing Strategy
Plan the fuzzing approach by deciding which parts of the API to target and how in-depth the fuzzing should be. Security engineers may choose to focus on specific endpoints, parameters
, or the overall API structure. Define the number of requests to send and the criteria for evaluating responses. The strategy should cover a broad scope without overwhelming the API with too much data at once.
5. Execute Fuzzing Tests
Run the fuzzing tests by sending crafted
payloads to the API and carefully monitoring the responses. Look for anomalies such as crashes, timeout errors, or incorrect responses that could indicate a vulnerability. Record all data for later analysis, as some issues may not be immediately apparent but could surface in post-test reviews.
6. Analyze Results
After completing the fuzzing, thoroughly analyze the results. Look for patterns, crashes
, or anomalies in the API’s behavior when exposed to unexpected inputs. This analysis will reveal weaknesses, vulnerabilities, or flaws in the API. Prioritize issues based on severity and potential impact on the API’s functionality or security.
7. Report and Remediate
Document the vulnerabilities found during fuzzing in a detailed report. Include the exact payloads used, the steps to reproduce
the issues, and the potential impact of each vulnerability. Collaborate with developers to address these flaws, ensuring that fixes
are tested and validated to prevent regression in future releases.
API Fuzzing Tools
API fuzzing relies on several powerful tools that security engineers and developers use to effectively test and secure APIs. Explore some of the most popular and efficient tools for API fuzzing below:
Akto
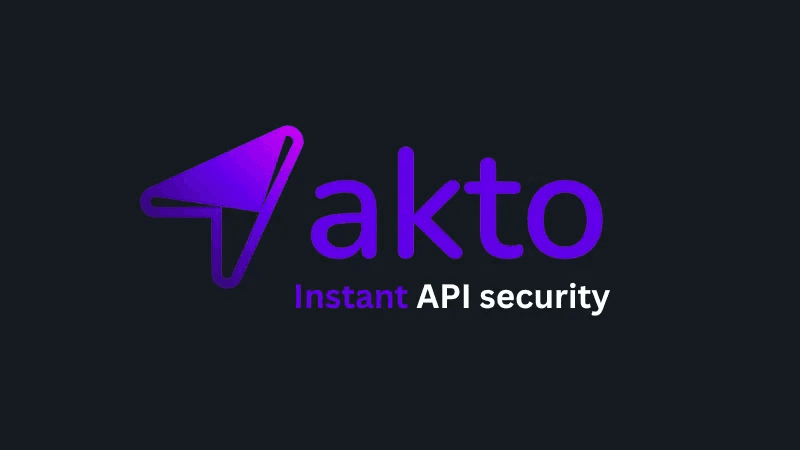
Akto serves as an API security platform designed to help organizations discover, monitor, and protect their APIs from vulnerabilities. It automates the process of identifying security flaws by running hundreds of built-in security tests, ensuring that APIs remain secure and compliant with industry standards.
Akto provides a specialized Lack of Resources and Rate Limiting (RL) testing template to detect vulnerabilities related to inadequate resource management and rate limiting in APIs. Without proper rate limiting, APIs become vulnerable to brute force attacks, denial-of-service (DoS), and other high-traffic abuses.
Kiterunner
Kiterunner performs traditional content discovery and brute-forces routes/endpoints in modern applications at lightning-fast speeds. Modern application frameworks like Flask, Rails, Express, and Django explicitly define routes that expect specific HTTP
methods, headers, parameters, and values.
The command to run Kiterunner looks like this:
In this command, routes.kite
serves as the wordlist for Kiterunner, and the scan
command scans all the endpoints based on the provided URL.
FFuf
Ffuf, an open-source fuzzing tool, swiftly explores web servers to discover hidden files, directories, and resources. It offers customizable fuzzing options, flexible output formats, and filters for refining results.
This ffuf
command performs fuzzing on a target URL by sending various inputs from a wordlist to discover hidden endpoints or vulnerabilities. The -c
option enables color-coded output for better readability, while -x http://<IP>:<PORT>
specifies a proxy server for routing all requests. The -r
flag prevents the tool from following redirects, focusing on initial responses from the server.
By using -fs 0
, the command filters out responses with a content size of 0, ignoring any empty responses. The -w <wordlist.txt>
option supplies the wordlist, replacing the FUZZ
keyword in the target URL (-u https://<target.com>/FUZZ
) with different inputs from the list. This approach tests how the target API reacts to varying inputs and potentially uncovers vulnerabilities or unprotected endpoints.
BurpSuite
BurpSuite serves as a powerful tool for API fuzzing and discovering hidden
endpoints. It offers a range of features that make it an excellent choice for API testing. The Intruder feature is particularly useful for crafting and automating customized attacks against an application's API, enabling the sending of different payloads and analyzing the results.
Burp Suite allows security testers to send a wide range of unexpected inputs to an API and monitor its responses. Its Intruder
feature lets testers automate the injection of multiple payloads into parameters to uncover vulnerabilities. Burp Suite also provides detailed feedback on server behavior, helping identify security flaws like injection attacks or improper input handling, making it an effective tool for discovering weaknesses in API security.
Final Thoughts
API fuzzing plays a crucial role in ensuring the robustness and security of an application’s API. By sending numerous invalid, unexpected, or random inputs and analyzing how the API responds, we can uncover potential bugs, security vulnerabilities, and unexpected behaviors. This proactive approach helps to address issues before they impact a production environment.
Akto, an API security platform, offers powerful capabilities for performing API fuzzing. It can automatically test your APIs for various vulnerabilities, helping you catch security flaws and performance issues early. With Akto, security engineers can integrate fuzz testing seamlessly into your API security workflows. To see how Akto can help secure your APIs, book a Akto demo today!
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.