Golang Fuzzing
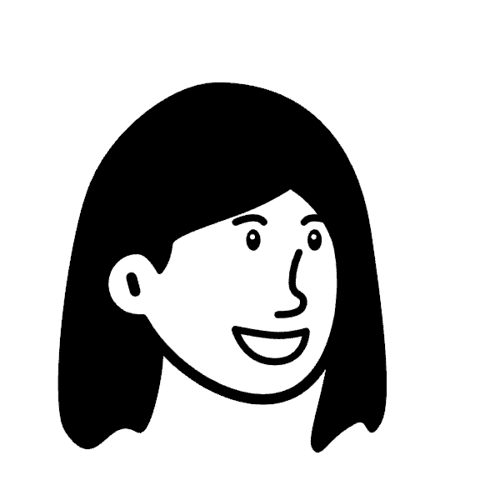
Golang fuzzing is a testing technique that automatically generates random or unexpected inputs for functions or programs written in Go. It aims to discover vulnerabilities, bugs, and unexpected behavior by analyzing how the program processes different inputs. This approach strengthens the reliability and security of Go applications by identifying edge cases and potential weaknesses.
This Blog explores fuzz testing in Golang, a powerful technique for uncovering bugs and security vulnerabilities in applications. It discusses its automation capabilities and benefits like enhanced code coverage and cost-effectiveness, and provides practical guidance on writing effective fuzz tests. This essential tool enables developers and security engineers to improve the reliability and security of their software.
What is Golang Fuzzing?
Golang fuzzing is an automated testing technique
in Go that generates random inputs to identify bugs, vulnerabilities, and abnormal behavior. This approach excels at discovering edge cases
and issues often missed by traditional testing. By providing unexpected inputs to functions, fuzzing in Go helps improve application stability and security.
This technique ensures that functions in Go programs
respond effectively to a wide range of input variations, helping developers detect issues early. By providing random or unexpected inputs, fuzzing strengthens application security
and stability, as it continuously probes for potential weaknesses.
Importance of Golang Fuzzing
Golang fuzzing is a vital technique in software testing that significantly enhances the security and reliability of applications. The following key aspects highlight its importance:
1. Automated Bug Detection
Golang fuzzing automates the process of detecting bugs by providing random or unexpected inputs to programs. This approach identifies functional defects
like crashes and security vulnerabilities such as buffer overflows
and injection flaws. It explores various inputs that standard testing may not cover, allowing developers to catch critical issues early in the development cycle. Automated bug detection
speeds up the identification of potential problems, reducing the risk of costly fixes later.
2. Enhanced Security Testing
Fuzzing is particularly valuable for security testing as it simulates potential attack scenarios. By feeding malicious or malformed data into applications, developers can discover vulnerabilities that could be exploited by attackers.
This proactive security measure ensures that threats are detected and mitigated before reaching production environments
, significantly reducing the risk of exploitation. This type of testing strengthens application defenses by exposing weak points
before they can be targeted.
3. Maximized Code Coverage
Modern fuzzers are designed to maximize code coverage
by testing as many execution paths as possible within the application. They generate thousands of test cases per second
, increasing the chances of identifying edge cases and hidden bugs. This comprehensive testing uncovers issues that traditional testing methods often overlook, especially in complex codebases
. By covering more code paths, fuzzing enhances the overall stability and security of the application.
4. Integration with Development Processes
With native fuzzing support introduced in Go 1.18
, developers can seamlessly integrate fuzz testing into their existing workflows. This integration simplifies the testing process, allowing fuzzing to become part of continuous integration and continuous delivery (CI/CD
) pipelines.
Continuous fuzzing provides rapid feedback on potential issues, enabling developers to resolve them quickly and efficiently. By embedding fuzzing into development processes
, teams can maintain higher code quality throughout the development lifecycle.
5. Cost-Effectiveness
Fuzzing identifies bugs early in the development process
, which significantly reduces the cost of fixing issues later in the software lifecycle. Catching defects during development prevents expensive downtime, security breaches, or patches
after release. By addressing issues early, fuzzing ensures more reliable software and reduces the risks associated with post-deployment failures
or vulnerabilities.
6. Simplicity and Accessibility
Golang’s built-in
fuzzing capabilities make it easy for developers of all skill levels to implement fuzz testing. The simple syntax
and structure of fuzz tests allow even those new to fuzzing to quickly get started
.
This accessibility encourages wider adoption of fuzz testing, fostering a culture of proactive bug and vulnerability detection
within development teams. With straightforward integration and ease of use, fuzzing becomes a natural addition to the software development toolkit
.
How to Write Fuzz Test?
Writing an effective fuzz test in Go requires a structured approach that encompasses several essential steps. Each step builds upon the last, resulting in a comprehensive fuzz testing strategy supported by detailed examples and explanations.
1. Create a Test File
Name fuzz test files with the suffix _test.go
to enable the Go testing tool to automatically identify these files as containing test cases. For instance, create a file named fuzz_test.go
to serve as the repository for all fuzzing functions. Organizing tests in this manner maintains clarity and streamlines the testing process, facilitating navigation and understanding of the test suite.
2. Define the Fuzz Function
Define the fuzz function using a name that starts with Fuzz
and include *testing.F
as the argument. This structure prepares the function for fuzz testing. For example, write a function like this:
Specify the initial inputs for the fuzzing process within this function, setting the stage for the fuzzing target. This clear naming convention and argument structure signal to the testing framework that this function is intended for fuzz testing, facilitating proper execution and analysis.
3. Add Fuzzing Inputs
Utilize f.Add(...)
to supply an initial seed input for the fuzzer. These seed inputs serve as foundational data points that guide the fuzzer in generating additional test cases. For example:
Providing specific seed inputs enables the fuzzer to explore a wider range of conditions within the program. This exploration reveals edge cases and unexpected behaviors, leading to the discovery of potential bugs or vulnerabilities. The more varied and representative the seed inputs are, the more effectively the fuzzer simulates real-world scenarios.
4. Implement the Fuzz Target
Next, pass a function to f.Fuzz(...)
that takes *testing.T
as its first argument, followed by other parameters representing the inputs to test. This structure allows the fuzzer to systematically assess how the program responds to randomly generated inputs. Here’s an example:
Implementing this step enables thorough testing of the program's response to various inputs. Systematic analysis of the responses uncovers hidden bugs or vulnerabilities that may not surface during standard testing practices, ultimately strengthening the robustness of the code.
5. Execute the Fuzz Test
Finally, execute the fuzz test by running the command go test -fuzz
in the terminal. This command instructs Go to initiate the process of generating and testing a multitude of input cases against the specified target function. Use the command like this:
This command initiates the fuzz testing process, systematically generating inputs based on the defined parameters and running them against the target function. As a result, valuable insights into how the code handles unexpected or malformed inputs emerge, further enhancing its resilience and reliability.
Golang Fuzzing Test Example
Here’s an example demonstrating fuzz testing for a function that sums integers in a slice:
Explanation of the Code
Here is the explanation for the code above:
Fuzz Function: The
FuzzSum
function initiates the fuzzing process. It defines theseed values
for the fuzzer and provides a target function that processes the inputs.Seed Inputs: The seed values added with
f.Add()
helps the fuzzer generate further inputs by expanding theinitial cases
. These values guide the fuzzer in exploring different paths in the program, increasing the likelihood ofuncovering hidden bugs
.Fuzz Target: Inside the
f.Fuzz()
method, theSum
function processes the generated inputs. The test checks for conditions such asnegative sums
, which should not occur when working with non-negative integers. This behavior validates the function's correctness across a range of inputs.
Running the Fuzz Test
To initiate the fuzzing process and evaluate the robustness of your code, use the following command for executing the fuzz test:
This command initiates the fuzzing process for the FuzzSum
function. Run all fuzz tests in the project by using:
This command ensures that every function starting with Fuzz
is executed. Fuzzing runs continuously, generating inputs until the test completes or encounters an error.
Tools for Golang Fuzz Testing
Fuzz testing in Golang has evolved with built-in support and a variety of external tools that enhance testing workflows. These tools enable automated testing by generating random inputs to uncover bugs, vulnerabilities, and edge cases that might otherwise go unnoticed.
1. Native Fuzzing Support (Go 1.18+)
Go introduced native fuzzing support in version 1.18
, making it easier to integrate fuzz testing into development
. It uses the *testing.F
type, streamlining the fuzzing process without requiring additional dependencies.
Key Features
Leverages Go's testing framework for seamless integration.
Uses coverage-guided fuzzing, which focuses on testing untested code paths.
Automatically generates random inputs based on initial seeds, improving input diversity.
Example Usage
Command to Run
Native fuzzing allows developers to identify edge cases
and ensure that code remains robust under a variety of input conditions, all within Go's built-in tooling
.
2. go-fuzz
The go-fuzz
tool is one of the most popular external libraries
for fuzz testing in Golang, providing coverage-guided fuzzing and a flexible corpus management system. It allows developers to test their programs under random inputs and discover subtle bugs or crashes.
Key Features
Works with Go's native fuzzing and
libFuzzer
for extended functionality.Utilizes a persistent corpus of inputs that can be
reused
across test runs, enhancing coverage over time.Provides detailed logging of
crashes
, coverage metrics, and input mutations, aiding in the debugging process.
Example Usage
Command to Run
The flexibility of go-fuzz
allows developers to customize their fuzzing setup while providing detailed insights into discovered issues.
3. Go Interface Fuzzer
The Go Interface Fuzzer is a specialized tool that automates fuzz
testing for interfaces in Golang
. It generates tests that compare the behavior of different implementations of an interface
under random inputs, ensuring consistent functionality.
The Go Interface Fuzzer offers several key features that enhance testing capabilities. It automatically generates testing functions to stress-test
various implementations of the same interface, ensuring consistent behavior under randomized inputs. This approach effectively reduces the risk of interface-related
bugs. Additionally, the tool simplifies the process of testing multiple implementations
by allowing developers to execute comprehensive tests with a single command
, streamlining the testing workflow and improving overall code quality.
Usage Example
The tool generates fuzzing functions like FuzzStoreWith
and FuzzStore
, which can then be run as part of the test suite, ensuring that implementations behave as expected under various input scenarios.
4. OSS-Fuzz
OSS-Fuzz is a fuzzing service provided by Google
for open-source projects. It offers continuous fuzzing, integrating directly into CI/CD pipelines
to automatically test code for vulnerabilities and crashes.
OSS-Fuzz offers several key features that enhance the fuzz testing process. It continuously runs fuzz tests, enabling early identification
of vulnerabilities in the development cycle. The platform provides comprehensive infrastructure support, including automated crash reports
, which streamlines the process of locating and fixing bugs. Additionally, OSS-Fuzz integrates seamlessly with open-source
projects, offering a scalable
platform that facilitates ongoing testing efforts.
5. Custom Seed Corpus Tools
Custom seed corpus tools enhance fuzzing by allowing developers to specify a variety of seed inputs that target specific edge cases
. These tools help create diverse and comprehensive sets of inputs, ensuring that fuzz tests cover a wide range of scenarios.
Custom seed corpus tools offer key features that enhance fuzz testing effectiveness. They allow developers to create seed files
targeting specific code areas or input variations, integrate seamlessly with fuzz testing frameworks to initiate tests with known edge cases
or critical input sets, and increase the chances of uncovering hidden bugs by directing the fuzzer with carefully crafted inputs. These capabilities collectively improve the efficiency and thoroughness of fuzz testing processes.
Challenges and Considerations in Golang Fuzzing
Golang fuzz testing presents several challenges and considerations that require careful attention for successful implementation. Understanding these challenges helps developers implement effective fuzz testing strategies.
1. Achieving Comprehensive Code Coverage
Achieving comprehensive code coverage during Golang fuzz
testing proves challenging, particularly for complex applications
with intricate control flows. Developers must design fuzzing inputs that effectively exercise all possible code paths
, which can be difficult in large systems. To improve coverage and uncover edge cases, teams should focus on proper input design and continuously refine test cases based on feedback and testing outcomes.
2. Managing Resource Constraints
Fuzz testing in Go often demands significant computational resources
, including memory
and processing power
, to process a large number of inputs. Managing these resource constraints is essential to prevent performance bottlenecks.
Effective strategies include optimizing hardware usage
, implementing parallel processing
, and employing optimization techniques. These approaches can scale fuzzing operations while minimizing resource strain.
3. Interpreting Fuzzing Results
Interpreting results generated by fuzzing tools can pose challenges due to the high volume
of potentially suspicious inputs. Developers must differentiate between genuine vulnerabilities and false positives,
requiring a careful and methodical review of results. Prioritizing vulnerabilities based on severity and filtering out false alarms streamlines this process, enabling teams to focus on addressing the most critical issues first.
4. Addressing Time Constraints
Fuzz testing can be time-consuming, particularly when conducting extensive campaigns
aimed at thorough code coverage. Developers must balance the need for comprehensive fuzzing with project deadlines and development timelines
.
To mitigate delays, teams can prioritize key areas
for fuzzing or run fuzz tests in parallel with other development activities. This approach ensures that fuzz testing becomes an integral part of the development process without causing significant disruptions.
5. Handling Complex Data Structures
Golang programs frequently work with complex data structures
, such as nested arrays, maps
, and structs. Designing fuzzing inputs that effectively test these structures requires thoughtful consideration and presents unique challenges
.
Fuzzing tools must generate inputs that interact meaningfully with these data structures
to uncover potential vulnerabilities in their processing. This process often involves developing specific input generation strategies tailored
to the structures being tested.
6. Integrating with Existing Testing Frameworks
Integrating fuzz testing into existing testing frameworks and development pipelines
can present logistical challenges. Developers must ensure seamless integration of fuzz testing with CI/CD
systems, version control repositories
, and issue-tracking tools. Proper integration helps streamline the fuzzing process and ensures that fuzz testing becomes a regular part of the development workflow
, enhancing overall software quality.
7. Ensuring Compatibility with External Dependencies
Many Golang applications rely on external dependencies
, adding complexity to fuzz testing. Ensuring compatibility with these libraries and effectively testing interactions between different components is critical.
Developers must account for external dependencies in their fuzzing campaigns to guarantee comprehensive testing of the entire system. This consideration involves assessing how different components interact and ensuring that tests cover these interactions thoroughly.
Final Thoughts
Golang fuzz testing significantly improves software quality and security. By employing a systematic approach, comprehensive planning, and the appropriate tools, developers can effectively integrate fuzz testing into the development process. As Golang gains popularity and its fuzz testing tools evolve, an increase in the adoption of fuzzing techniques in testing strategies is expected, resulting in the production of robust and reliable software applications.
Akto, an API security platform, offers powerful capabilities for performing API fuzzing. It can automatically test the APIs for various vulnerabilities, helping security engineers to catch security flaws and performance issues early. With Akto, security engineers can integrate fuzz testing seamlessly into the API security workflows. To see how Akto can help secure the APIs, book a Akto demo today!
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.