API Security Checklist
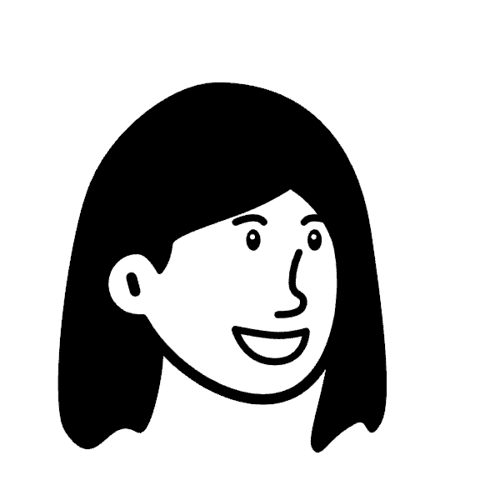
Insha
Oct 4, 2024
The API Security Checklist outlines the key practices and measures that organizations must implement to secure APIs against vulnerabilities and threats. It includes steps to validate authentication and authorization mechanisms, ensure proper input validation, implement rate limiting, and protect sensitive data.
This blog will guide you through the essential steps to safeguard APIs effectively. You'll learn how to implement robust authentication mechanisms, ensure secure data transmission, and set up monitoring systems to detect potential threats.
What is an API Security Checklist?
An API Security Checklist is a comprehensive list of best practices and security measures that developers, testers, and security teams use to secure an API. It covers all critical aspects of API security, such as authentication, authorization, data validation, rate limiting, logging, and error handling.
The checklist helps developers and security engineers develop, test, and deploy APIs securely to prevent common vulnerabilities like data breaches, unauthorized access, and misuse by attackers. By following an API security checklist, organizations can proactively strengthen their APIs against potential security risks.
8-Step API Security Checklist
The API security checklist emphasizes the importance of regularly testing APIs for security flaws
, monitoring for unusual activities, and keeping documentation up to date. By following this checklist, organizations can systematically identify and address potential security issues, ensuring their APIs remain secure and reliable.
1. Authentication and Authorization
Strong Authentication Methods
Use strong authentication methods to verify the identity of users and applications accessing the APIs. These include:
OAuth2.0: OAuth 2.0 allows users to give applications limited access to their data without needing to share their passwords. Instead of providing their login credentials, users authorize access by granting a specific set of permissions, such as reading their profile information or accessing certain files. It issues tokens to handle permissions, allowing applications to perform only the actions that users have explicitly authorized. By separating credentials from access, OAuth2.0 ensures that users maintain control over their data while enhancing security and minimizing the risk of unauthorized access.
How to Implement
Register the application with an
OAuth2.0
provider (e.g., Google, Facebook).Implement the OAuth2.0 flow in the application to obtain access tokens.
Use these tokens to authenticate API requests.
Example: When a user logs in using their Google account, redirect them to Google’s OAuth2.0 authorization endpoint. Upon successful login, Google provides an access token, which the application uses to access the user’s data.
JWT (JSON Web Tokens): JWTs (
JSON Web Tokens
) securely transmit information between parties as a compact JSON object. They contain a payload with user data and a digital signature to verify the token's authenticity. This signature verifies that the data has not been tampered with, providing a reliable method for securely sharing information across different systems or applications. JWTs are often used in authentication and authorization processes, enabling secure, stateless communication between client and server.How to Implement
Generate a JWT when a user logs in.
Include the JWT in the Authorization header of API requests.
On the server, verify the JWT’s signature and extract the user information.
Example: After a user logs in, your server generates a JWT with their user ID and role. The client includes this token in subsequent requests. The server verifies the token and uses the user ID and role to grant or deny access.
Mutual TLS (Transport Layer Security): In mutual TLS (T
ransport Layer Security
), the client and server use certificates to authenticate each other. The client presents its certificate to prove its identity, while the server verifies it before establishing a connection. At the same time, the server sends its certificate to the client, which also performs a verification. Thistwo-way authentication
ensures that both parties are genuine, enhancing security by preventing unauthorized access andman-in-the-middle attacks
.How to Implement
Generate and distribute client and server certificates.
Configure the server to require client certificates for authentication.
Validate the client certificate on each request.
Example: Your API server requires a client certificate. The client application presents its certificate when connecting. The server verifies the certificate against a trusted CA, ensuring secure, mutual authentication.
Role-Based Access Control (RBAC)
Implementing Role-Based Access Control
(RBAC) is essential for managing who can access different parts of the API. To do this, start by defining roles based on job functions, such as admin, user, or guest. For example, an organization might create roles like Admin, who has full access, Editor, who can view and edit data, and Viewer, who can only view data.
To enforce RBAC, ensure that the API verifies the user’s role before granting access to sensitive information or actions. Implement this by using middleware or access control checks in API endpoints. The API should check the user’s role and compare it against the required permissions for that endpoint, denying access if the user does not have the necessary permissions.
For example, before allowing a request to delete a resource, the server checks if the user holds the Admin role. If not, it responds with a "403 Forbidden
" message, effectively blocking unauthorized actions.
2. Input Validation and Output Encoding
To prevent injection attacks, such as SQL or command injection, always validate all incoming data. Start by checking that the data type matches the expected type, like numbers, strings, or dates. Implement whitelisting
to allow only known good values and reject any unexpected input. Limit the length and enforce specific formats for data, such as email addresses or phone numbers. For example, if a user submits their age, confirm that it is a number within a reasonable range.
To prevent Cross-Site Scripting (XSS) attacks, encode data before sending it to the client. Escape special characters, such as <, >, &, and ", by converting them to their HTML entity equivalents, like <, >, &, and. “Use built-in functions in the framework or programming language to handle encoding automatically.
Example: If you display a user’s name on a webpage, ensure that any special characters are escaped so attackers cannot execute malicious scripts.
This JavaScript code validates and secures user input to prevent attacks like Cross-Site Scripting (XSS). It first checks whether the input contains only alphanumeric characters using a regular expression. If the input is valid, it then encodes special characters (&
, <
, >
, "
, '
) into their HTML entity equivalents to ensure that any malicious scripts are displayed as plain text rather than executed.
If the input fails validation, it logs "Invalid input" to the console. In the given example, the input <script>alert('XSS');</script>
would be deemed invalid due to non-alphanumeric characters.
3. Rate Limiting and Throttling
Rate Limiting
Rate limiting is a crucial technique to control how often users or applications can make requests to the API within a specific time frame. By ensuring that all users have fair access to your API, rate limiting also helps maintain the performance and reliability of the services.
Set Limits: Determine the maximum number of requests each user or IP can make, such as
100 requests per minute, hour, or day
. Adjust these limits based on the API’s expected usage patterns and traffic volumes.Use Tools and Libraries: Many API frameworks offer built-in solutions for implementing rate limiting. For example, in a Node.js environment, an organization can use the
express-rate-limit
middleware to define and enforce request limits effortlessly.Monitor and Adjust: Monitor API usage patterns regularly to identify unusual activity or potential abuse. Adjust rate limits to prevent malicious behavior while ensuring legitimate traffic flows smoothly. Analyze traffic trends to balance security with accessibility. Use analytics to understand user behavior and make informed rate-limiting decisions.
Example: Implement a limit where each user can only make 100 requests per hour. If a user exceeds this limit, the API responds with an HTTP 429 (Too Many Requests)
status code, informing them to slow down or try again later.
This code sets up rate limiting for an Express.js application. It limits each IP address to 100 requests per hour and returns a message, "Too many requests from this IP, please try again later
," If the limit is exceeded, the app.use(limiter)
line applies this rate limit to all incoming requests.
Throttling
Throttling maintains API performance and security by slowing down or blocking excessive requests, ensuring the API remains responsive and accessible to all users. To implement throttling, organizations should use gradual throttling
, in which the response rate for users who make too many requests is slowly reduced, introducing delays instead of outright blocking them. Alternatively, security teams can block requests that exceed a specific threshold to prevent potential abuse or attacks.
Example: This example shows how the “express-slow-down” middleware is used to throttle requests.
This code uses the express-slow-down
middleware to throttle requests to an Express.js application. It allows each IP address to make up to 50 requests per hour without delay. After the 50th request, the code begins adding a 500-millisecond
delay to each additional request within the same hour. The app.use(speedLimiter)
line applies this slowing mechanism to all incoming requests, gradually reducing the speed for users who exceed the allowed limit.
4. Secure Data Transmission
Securing data transmission is critical for protecting sensitive information, such as personal data and login credentials, from interception by attackers. Organizations should always use HTTPS (Hypertext Transfer Protocol Secure
) to encrypt data transmitted between clients and servers. HTTPS establishes a secure connection, ensuring that data remains confidential and protected from eavesdropping or tampering.
How to Implement
1. Always Use HTTPS
HTTPS encrypts the communication between the client and the server, preventing unauthorized access to sensitive information. Follow these steps to implement HTTPS:
Obtain an SSL/TLS Certificate
Acquire an
SSL/TLS
certificate from a trustedCertificate Authority
(CA). This certificate validates your website’s identity and enables encrypted connections. Free options like Let’s Encrypt provide SSL/TLS certificates that are easy to set up and maintain.Configure Your Server to Use HTTPS
Set up your web server, such as Apache or Nginx, to use HTTPS. Refer to the server documentation or guides to correctly configure SSL/TLS. This setup includes installing the certificate and specifying HTTPS as the protocol for secure connections.
Redirect All HTTP Traffic to HTTPS
Redirect all incoming HTTP requests to HTTPS to enforce secure connections. This ensures users always connect through an encrypted channel, protecting their data. Set up automatic redirects to eliminate insecure access points.
Example
This example shows how to configure an Nginx server to redirect HTTP traffic to HTTPS, ensuring secure connections by encrypting data between the client and server.
The code starts with a server block listening on port 80 for HTTP traffic and uses a 301
redirect to permanently redirect all HTTP requests to HTTPS. The second server block listens on port 443 for HTTPS connections and is configured with the ssl_certificate
and ssl_certificate_key
directives, which specify the SSL/TLS certificate and private key for encrypting data.
The location
directive checks for requested files or directories and serves them if available, returning a 404 error if not. This configuration forces all traffic to use HTTPS, ensuring secure, encrypted communication between clients and the server.
2. Enforce SSL/TLS to Prevent Attacks
SSL/TLS encryption prevents MITM
attacks by establishing secure communication channels. Here are key practices to enforce SSL/TLS:
Use Strong Protocols and Ciphers
Disable outdated protocols like
SSL 2.0 and 3.0
, which are vulnerable to attacks, and enable only secure versions, such asTLS 1.2 or 1.3
. Choose strong cipher suites that provide robust encryption to protect the integrity and confidentiality of data. Configuring the server to use strong protocols and ciphers enhances security.Regularly Update and Renew Certificates
Keep your SSL/TLS certificates up-to-date and renew them before they expire. Expired certificates can cause security warnings in users’ browsers and potentially expose the data to risks. Regularly check the certificates’ validity and renew them promptly to maintain trust and secure communication.
HTTP Strict Transport Security (HSTS)
Security engineers should configure the web server to use
HTTP Strict Transport Security
(HSTS) headers, which tell browsers that the site should only be accessed using HTTPS. HSTS prevents protocol downgrade attacks by enforcingHTTPS-only
connections. This mechanism protects users even if they initially attempt to connect over HTTP.Example
This example configures an Nginx server to use strong SSL/TLS protocols and ciphers while enforcing HTTP Strict Transport Security (HSTS) to keep all communication secure.
The code configures an Nginx server to use SSL/TLS for secure communication by specifying the certificate and key files. It enables only strong TLS protocols (TLS 1.2 and 1.3) and sets strong ciphers for encryption. The add_header
directive enforces HSTS, ensuring that all connections use HTTPS. The location block handles requests and serves files securely, returning a 404 error
if it does not find the file.
5. Logging and Monitoring
Logging
Logging all API activity helps organizations track usage, identify issues, and respond to security incidents. Logs provide a record of all interactions with the API, which is crucial for debugging, auditing, and investigating suspicious behavior
.
How to Implement
Log Every Request and Response: Capture details like
timestamps
, IP addresses, accessed endpoints,request payloads
, and response statuses for every API interaction. Use this information to track activity and quickly identify any suspicious behavior.Secure Log Storage: Store logs in a secure location and restrict access to only authorized personnel. Apply encryption and strong access controls to prevent unauthorized access and tampering.
Implement Logging Frameworks: Select logging frameworks or services that integrate well with the API, like
Log4j for Java
,Winston for Node.js
, or theELK stack (Elasticsearch, Logstash, Kibana)
for centralized logging. These tools allow organizations to efficiently collect, manage, and analyze log data.
Example: In Node.js, use the morgan
middleware to log HTTP requests.
This code sets up a basic Express.js server and uses the morgan
middleware to log HTTP requests.
It first requires the necessary modules:
express
to create the server andmorgan
to handle request logging.The code creates an instance of an Express application with
const app = express()
.It applies the
morgan
middleware to log all HTTP requests in the "combined" format, which includes detailed information such as the remote IP, request method, URL, HTTP status code, response time, and more.The code defines a route for the root URL (
'/'
). When a client makes a GET request to this route, the server responds with "Hello, World!".Finally, it starts the server on port 3000 and prints "
Server is running on port 3000
" to the console when the server successfully starts.
Monitor for Anomalies
Monitoring systems allow organizations to detect unusual patterns or potential security incidents as they happen, so the organization can respond immediately and reduce the impact on the system and users.
How to Implement
Set Up Real-Time Monitoring: Deploy monitoring tools such as
Prometheus
, Grafana, orDatadog
to track API metrics like request rates, error rates, and latency in real time. These tools help organizations understand how the API performs under different conditions and detect any anomalies that may indicate security threats or performance issues.Define Alerts for Anomalies: Configure alerts to trigger notifications whenever the system detects unusual activities, such as a surge in traffic, multiple failed login attempts, or access requests from unknown IP addresses. Set specific thresholds for these alerts to quickly identify potential attacks or unauthorized activities.
Analyze Logs and Metrics: Regularly examine logs and analyze collected metrics to uncover trends or patterns that could signal security risks or performance bottlenecks. Use this data to improve organization security measures and optimize API performance, ensuring the system remains secure and efficient over time.
Use the Akto Prometheus template to find exposed Prometheus endpoints
6. API Gateway and Firewall
An API gateway secures APIs by acting as a centralized control point
for all API traffic. It allows organizations to manage requests, enforce security policies, authenticate users, apply rate limiting, and log activities, all from a single location.
When traffic reaches the gateway, it directs each request to the appropriate backend service while verifying user identities and ensuring that only authorized users access the resources. This setup helps developers and security engineers implement consistent security measures, such as requiring secure tokens for authentication and validating user permissions across all services.
A firewall adds another layer of security by filtering both incoming and outgoing traffic based on pre-defined security rules. It inspects each request and blocks unauthorized access, detecting and preventing potential threats like SQL injection, cross-site scripting (XSS), and other malicious activities before they reach the API. The firewall also identifies and stops abnormal traffic patterns that could indicate attacks, such as Distributed Denial of Service
(DDoS) attempts.
Example
AWS API Gateway provides a fully managed service for creating, deploying, and managing APIs. It integrates with AWS Lambda, EC2, and other services to handle traffic, security, and monitoring.
This code configures an AWS API Gateway to set up a REST API that integrates with an AWS Lambda function.
It defines a resource named
MyApi
of typeAWS::ApiGateway::RestApi
, which creates a new API Gateway instance named "MyApi" with the description "My API Gateway."The
MyApiResource
resource creates a new API endpoint. It specifies theParentId
using the root resource ID of theMyApi
instance and setsPathPart
to "{proxy+}", which creates a proxy resource that captures all paths.The
MyApiMethod
resource defines an HTTPGET
method for theMyApiResource
. It sets theAuthorizationType
to "NONE," allowing access without authentication.For this method, it specifies integration with an AWS Lambda function using the
AWS_PROXY
type. TheIntegrationHttpMethod
is set toPOST
to invoke the Lambda function. TheUri
parameter provides the ARN of the Lambda function, specifying the function to be executed when a request is received.
Web Application Firewall (WAF)
A Web Application Firewall (WAF) protects the APIs by filtering and monitoring HTTP requests. It helps organizations protect against common web exploits and attack patterns, such as SQL injection, cross-site scripting (XSS), and other threats.
How a WAF Works
Filters Requests: A WAF inspects each incoming request and blocks any that match known attack patterns or violate predefined security rules. This helps prevent malicious activities like SQL injection or cross-site scripting (XSS).
Monitors in Real-Time: It continuously monitors traffic for any suspicious activity, immediately detecting potential threats. It provides real-time alerts and logs to help organizations respond quickly to security incidents.
Enforces Custom Rules: A WAF (Web Application Firewall) enables organizations to define
custom rules
tailored to their specific security needs, effectively protecting against targeted threats. This flexibility allows organizations to fine-tune their security measures based on the unique requirements of their applications, ensuring a more robust and effective defense.
Example
This example demonstrates how to configure an AWS WAF (Web Application Firewall) to protect against SQL injection attacks by defining a WebACL (Web Access Control List
) with specific rules that block malicious requests.
This code sets up an AWS WAF (Web Application Firewall) configuration to protect the application from SQL injection attacks.
It creates a
WebACL
resource of typeAWS::WAFv2::WebACL
, defining a Web Access Control List (Web ACL) for the application. The configuration sets theDefaultAction
to allow all traffic unless a rule explicitly blocks it.The configuration defines a rule named
SQLInjectionRule
with a priority of 1, meaning the system evaluates it first. This rule blocks requests that contain a specific SQL injection pattern(' OR '1'='1)
, which attackers commonly use in SQL injection attacks.It uses a
ByteMatchStatement
to specify the conditions for blocking requests. TheFieldToMatch
is set to theQueryString
of incoming requests, and thePositionalConstraint
is set toCONTAINS
, meaning it looks for the specified string anywhere in the query string.The
VisibilityConfig
for the rule enables CloudWatch metrics (CloudWatchMetricsEnabled: true
) to monitor and collect data on how often this rule triggers. It sets aMetricName
for the rule as "SQLInjectionRule" and allows sampled request logging (SampledRequestsEnabled: true
).The
VisibilityConfig
for the entireWebACL
enables CloudWatch metrics to monitor overall web traffic, setting "WebACL" as the metric name and enabling sampled requests logging.
7. Error Handling
Secure error messages prevent attackers from gaining insights into the application's internal workings, which they could exploit to launch further attacks. Handling errors securely ensures that users receive useful feedback without revealing sensitive information.
How to Implement
Disable Stack Traces in Production: Turn off
stack traces
and detailed error information in production environments to prevent exposing sensitive details. Keep detailed logs of errors internally for debugging purposes.Sanitize Error Outputs: Remove technical details from error messages before showing them to users. Ensure that the messages are generic and do not reveal any information that could help an attacker exploit vulnerabilities.
Implement Centralized Error Handling: Use centralized error handling middleware in the application to manage all errors consistently. This middleware should catch and handle errors globally, providing uniform error messages to users and logging necessary details for developers and security engineers.
Example
This example sets up a basic Express.js server that logs detailed error information internally and sends a generic error message to the user.
This code sets up an Express.js server that listens on port 3000 and handles requests to the root URL ('/'
).
It creates an Express application using
express()
and defines a route for the root URL ('/'
). When a user sends a GET request to the root URL, the server attempts to execute the code inside the route.Inside the route, the code deliberately simulates an error by throwing an exception with the message "Something went wrong!"
When the error occurs, the
catch
block captures it. The server logs the error details to the console for internal debugging usingconsole.error(error)
.After logging the error, the server sends a generic error message ("An error occurred. Please try again later.") back to the user with an HTTP status code of 500, indicating an internal server error.
The server starts listening on port 3000, and it prints "
Server is running on port 3000
" to the console once it starts successfully.
8. Regular Security Testing and Automation
Regular security testing and automation are essential practices for maintaining a strong security posture in API. By proactively identifying and addressing vulnerabilities, organizations can reduce the risk of exploitation and ensure their API remains secure against evolving threats. Implementing both manual and automated security testing helps catch issues early in the development process, reducing the cost and effort needed to fix them later.
How to Implement
Conduct Vulnerability Scans
Use automated tools like OWASP ZAP,
Nessus
, or Burp Suite to scan for common vulnerabilities such as SQL injection, cross-site scripting (XSS), and insecure configurations. These tools help identify weaknesses in the API that attackers could exploit.For Example, schedule monthly vulnerability scans to regularly check the API for new vulnerabilities.
Perform Penetration Testing
Conduct regular penetration tests by hiring ethical hackers to simulate real-world attacks. This method allows organizations to discover potential security gaps and understand how an attacker might exploit them.
For Example, schedule annual penetration tests to ensure that the security teams identifies and addresses any new vulnerabilities promptly.
Integrate Security Tools into Your CI/CD Pipeline
Incorporate security testing tools like Snyk, Checkmarx, and SonarQube into organization Continuous Integration/Continuous Deployment (CI/CD) pipeline. These tools automatically scan your code for vulnerabilities during the build process, ensuring that security checks are performed consistently and frequently.
For Example, use Jenkins to integrate OWASP ZAP for Dynamic Application Security Testing (DAST) and SonarQube for Static Application Security Testing (SAST).
Add Security Testing Stages to the Pipeline
Include stages for security testing in your pipeline, such as:
SAST: Analyze source code for known vulnerabilities without running the program.
DAST: Test the running application for security vulnerabilities by simulating attacks.
Software Composition Analysis (SCA): Check for vulnerabilities in open-source components and libraries used in your API.
Example: Configure the pipeline to run these tests on every code commit to detect vulnerabilities early.
Fail the Build on High-Risk Issues
Set the CI/CD pipeline to fail builds if it detects high-risk vulnerabilities. This approach ensures that only secure code is deployed to production, preventing insecure releases.
For Example, configure
Jenkins
to halt the deployment process if OWASP ZAP or SonarQube identifies critical security issues.
Example Here is an example of a Jenkins pipeline configuration (in Groovy) that includes automated security testing:

Build Stage: The
Build
stage compiles and builds the application. This step ensures that the application is ready for further testing.Static Code Analysis Stage: The
Static Code Analysis
stage runs tools like SonarQube to analyze the source code for vulnerabilities without executing it. This stage helps detect coding flaws that could lead to security risks.Dynamic Security Testing Stage: The
Dynamic Security Testing
stage uses tools like OWASP ZAP to simulate real-world attacks on the running application. It checks for vulnerabilities that only appear during runtime, such as XSS or SQL injection.Post Actions: The
post
section contains actions that run after the pipeline completes. Thealways
step executes cleanup actions regardless of success or failure, while thefailure
step triggers when the pipeline fails, notifying the team of security issues that caused the failure.
Final Thoughts
Organizations actively secure their APIs by regularly reviewing and updating security practices. They validate all inputs and outputs to prevent injection attacks, implement rate limiting
to control traffic, and always use HTTPS to ensure secure data transmission. Detailed logs of API activity enable organizations to quickly detect and respond to potential threats, while proper error handling prevents the exposure of sensitive information.
Akto's powerful platform enhances API security. Akto automates API security testing and provides real-time monitoring and automated checks to help organizations identify and mitigate risks effectively. Organizations can explore how Akto's solutions safeguard their APIs and strengthen their overall security posture by booking a demo.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.