Security Misconfiguration in API
Security misconfiguration occurs when improper or default settings leave APIs vulnerable to attacks, potentially leading to unauthorized access or data breaches.
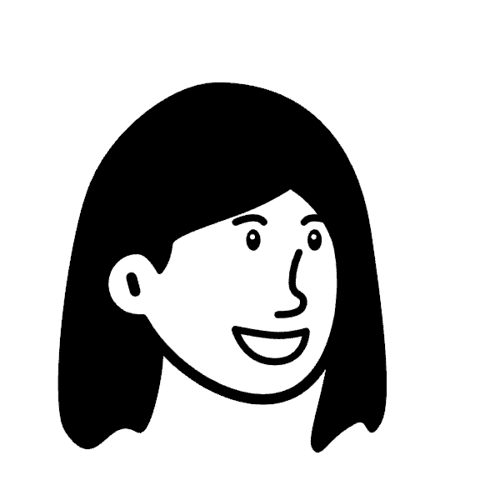
Security misconfiguration happens when security settings are incorrectly applied or left in an insecure state. This often occurs when default settings are not changed, unused features remain active, or inconsistent configurations are applied across different systems.
These missteps create vulnerabilities that attackers can exploit, leading to unauthorized access or data breaches. Regular checks and updates to configurations are essential to avoid these risks and maintain strong security defenses.
In this blog, you will learn about API security misconfigurations, explore vulnerabilities, discover prevention strategies, and find tools to strengthen the organization's API security posture.
What is Security Misconfiguration?
Security misconfiguration in APIs occurs when settings and configurations are not properly set up, leaving the API vulnerable to attacks. This can include using default settings, not disabling unnecessary features, or failing to update configurations over time.
This can lead to various vulnerabilities, making it easier for attackers to exploit the API. Setting up and maintaining API configurations is essential to prevent security breaches and protect sensitive information.
Common Security Misconfigurations in APIs
Security misconfigurations in APIs expose critical vulnerabilities that attackers can exploit, compromising data integrity and system security. Let's explore the most common types of misconfigurations:
Exposed API Endpoints
Leaving API endpoints exposed to the public can allow attackers to access sensitive data
or perform unauthorized actions. By not securing these endpoints, organizations increase the risk of malicious activities. Implementing proper authentication and access controls for each endpoint helps protect against unauthorized access.
Default Configurations
APIs often come with default settings, such as open ports, default credentials, or weak security settings. Leaving these configurations unchanged can create significant vulnerabilities, giving hackers easy access to your system.
Default configurations may also include unnecessary features or permissions that broaden the attack surface. It is critical to review and customize these settings immediately after deployment to meet the organization’s specific security requirements.
Lack of Rate Limiting
Without rate limiting, APIs are vulnerable to abuse from attackers who can flood the system with excessive requests, leading to service disruptions, slowdowns, or even complete outages. Attackers may also exploit this to conduct brute force attacks
or search for vulnerabilities by sending an overwhelming number of requests.
Implementing rate limiting allows security teams to control the flow of traffic, capping the number of requests a user or system can make within a set time frame. This helps maintain API performance and prevents malicious users from overloading the system.
Verbose Error Messages
When APIs return detailed error messages, they expose more than just the issue—they often reveal critical information about the system’s internal structure, such as database names, server configurations
, or even code snippets.
Attackers can leverage this information to craft more precise and effective attacks, targeting specific weaknesses in the organization's system. By leaving verbose error messages unconfigured, organizations unintentionally assist malicious users in gathering the data they need to exploit vulnerabilities.
Missing Security Headers
When APIs lack proper security headers, they leave the system exposed to a range of attacks, including cross-site scripting (XSS), clickjacking, and man-in-the-middle attacks. Security headers like Content Security Policy
(CSP) help control what resources can be loaded on a page, preventing attackers from injecting malicious scripts.
Similarly, HTTP Strict Transport Security
(HSTS) ensures that browsers only interact with the server over secure HTTPS connections, reducing the risk of data interception. Without these critical headers, attackers can exploit vulnerabilities to hijack user sessions, steal sensitive information, or manipulate data.
Lack of Proper Authentication and Authorization
When APIs lack proper authentication and authorization mechanisms, they fail to verify who is accessing them and what actions the user is permitted to perform
. This opens the door for unauthorized users to access, modify, or delete sensitive data, leading to potential data breaches and security risks.
For example, an API that does not enforce strict user authentication may allow anyone to access it without verifying their identity, enabling malicious actors to view or alter confidential information.
Impact of Security Misconfiguration
Security misconfigurations in APIs can have severe consequences for organizations, impacting data integrity, system vulnerabilities, and overall business operations.
Increased Risk of Data Breaches
Security misconfigurations significantly increase the risk of data breaches by exposing sensitive data to potential attackers. When organizations fail to properly configure security settings, they create gaps in their defenses, making it easier for hackers to exploit vulnerabilities.
These misconfigurations can lead to the leakage of critical information
such as customer details, financial data, or internal records. Attackers can then use this exposed data for malicious purposes, including identity theft or financial fraud.
Vulnerable to Attacks
Misconfigured APIs and systems provide attackers with easy entry points to exploit. Hackers can capitalize on weak security settings, such as open ports, default credentials, or outdated software, to infiltrate systems and launch damaging attacks.
These vulnerabilities allow attackers to execute tactics like SQL injection, cross-site scripting (XSS), or even full-scale denial of service
(DoS) attacks that can disrupt services or steal sensitive data. By failing to secure APIs properly, organizations allow malicious actors to bypass protections, escalate privileges, or cause system outages.
Non-Compliance with Regulations
Security misconfigurations can directly cause organizations to fall out of compliance with critical industry regulations like GDPR, HIPAA
, or PCI-DSS. Regulatory bodies mandate strict security controls to protect sensitive data, and when companies fail to properly configure their systems, they risk violating these standards.
For example, leaving sensitive data unencrypted or failing to enforce strong access controls
can breach GDPR’s data protection rules, exposing personal information. Non-compliance doesn't just open the door to hefty fines—it also damages the organization's reputation, eroding trust with customers and partners.
Operational Disruptions
Misconfigured APIs can lead to performance issues or system downtime, disrupting critical operations. These disruptions slow down workflows, reducing overall productivity and delaying key business processes. As a result, the organization may face financial losses due to inefficiencies and the cost of fixing the issues.
Loss of Customer Trust
Security misconfigurations that lead to data breaches or cyberattacks severely damage customer trust. When clients share their personal or financial information, they expect it to be protected. If an organization fails to secure its data, customers may feel betrayed, and their confidence in the organization's ability to safeguard sensitive information
erodes. This loss of trust often leads to negative publicity, causing potential customers to turn to competitors.
Increased Maintenance Costs
Fixing misconfigurations after a breach or attack often requires significant time and resources. Organizations may face increased maintenance costs due to the need for emergency patching, incident response, and remediation efforts. Regular audits
and proactive configurations help avoid these expenses.
Security Misconfiguration Examples
Let's explore a common security misconfiguration example that highlights the potential risks and vulnerabilities in API implementations.
Misconfigured CORS Policy
A common security misconfiguration involves setting a permissive Cross-Origin Resource Sharing
(CORS) policy, which allows external domains to make requests to the API or web server. Browsers implement CORS as a security feature to prevent unauthorized external websites from interacting with resources on the organization’s server. Misconfiguring CORS can open the system to cross-origin attacks, exposing sensitive data to malicious websites.
Code Example (in Express.js)
What This Code Is Doing?
Permissive CORS Policy
The line
app.use(cors())
enables CORS for all domains, meaning any external website can send requests to the server and access the data. While this might be useful during development, it is extremely dangerous in production environments. It exposes the API to any website, potentially leading to cross-origin attacks where malicious websites steal sensitive data or perform unauthorized actions on behalf of users.No Access Control
Since the CORS policy allows all domains, the policy has no restrictions on who can access the API. This means attackers can trick users into making
unintended requests
to the API, exposing sensitive information or executing actions without their knowledge.
Risks of Permissive CORS
Data Exposure
Permissive CORS allows malicious websites to send requests to the API without proper authorization, easily accessing sensitive data. Once attackers obtain this data, they can expose it to unauthorized users or sell it to other malicious entities.
This lack of control over who can access the API raises the chances of data leaks, putting customer information, intellectual property, and internal business data at risk. Attackers exploit the permissive policy to repeatedly access sensitive information, leaving the organization vulnerable to constant data breaches.
Cross-Site Request Forgery (CSRF) Attacks
In a permissive CORS environment, attackers can execute CSRF
attacks by tricking users into unknowingly submitting requests to the API. These unauthorized requests can lead to dangerous consequences, such as unauthorized fund transfers, data deletions, or changes to user profiles.
Because the attacker can impersonate a legitimate user, the API might process these requests as if they were authorized, leading to account takeovers or the compromise of user data. This attack can happen without the user's knowledge, leaving the victim and the organization unaware of the manipulation until significant damage is done.
Preventing Security Misconfigurations
To avoid this issue, security teams need to restrict CORS to trusted domains and only allow specific methods
and headers. Always configure CORS carefully to avoid exposing the organization’s system to security risks.
Revised Secure Configuration
What This Revised Code Is Doing
Restricted CORS Policy
The
corsOptions
object restricts access to the API, allowing only requests fromhttps://trusted-domain.com
. This prevents unauthorized external websites from making requests to the API. By specifying trusted domains, security teams minimize the risk of exposing sensitive data to attackers.Limited Methods and Headers
The
methods
andallowedHeaders
properties restrict which HTTP methods and headers can be used when making requests to the API. This ensures that only specific, necessary actions are allowed, further reducing the risk of exploitation.
Leaving CORS policies wide open can lead to serious security vulnerabilities, such as data exposure and cross-site request forgery (CSRF) attacks. By carefully configuring CORS to only allow trusted domains and limiting methods and headers, organizations can significantly reduce the risk of cross-origin attacks. Regularly reviewing and auditing CORS settings ensures that the API remains secure from unauthorized access and manipulation.
How Akto Detect Security Misconfigurations
Akto empowers organizations to detect and prevent security misconfiguration in their APIs through its comprehensive suite of tools and automated processes.
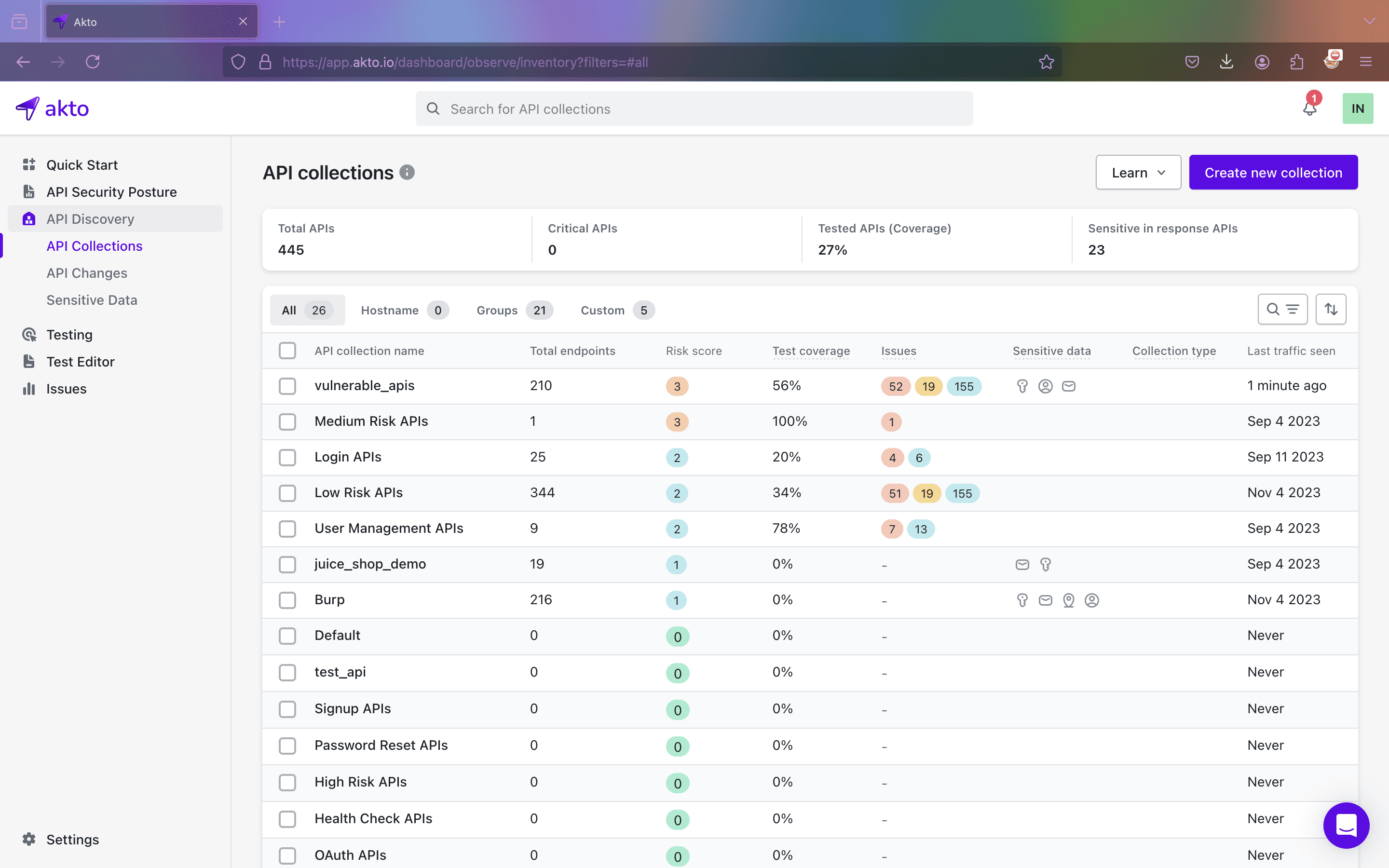
Akto automatically discovers and monitors APIs in real-time across various formats, including REST, GraphQL, gRPC, and JSONP. The system eliminates the need to manually update the swagger file by creating an up-to-date swagger file and proactively alerting security teams about new or changed APIs. This enables efficient management of API security risks while ensuring visibility into both external and internal APIs.
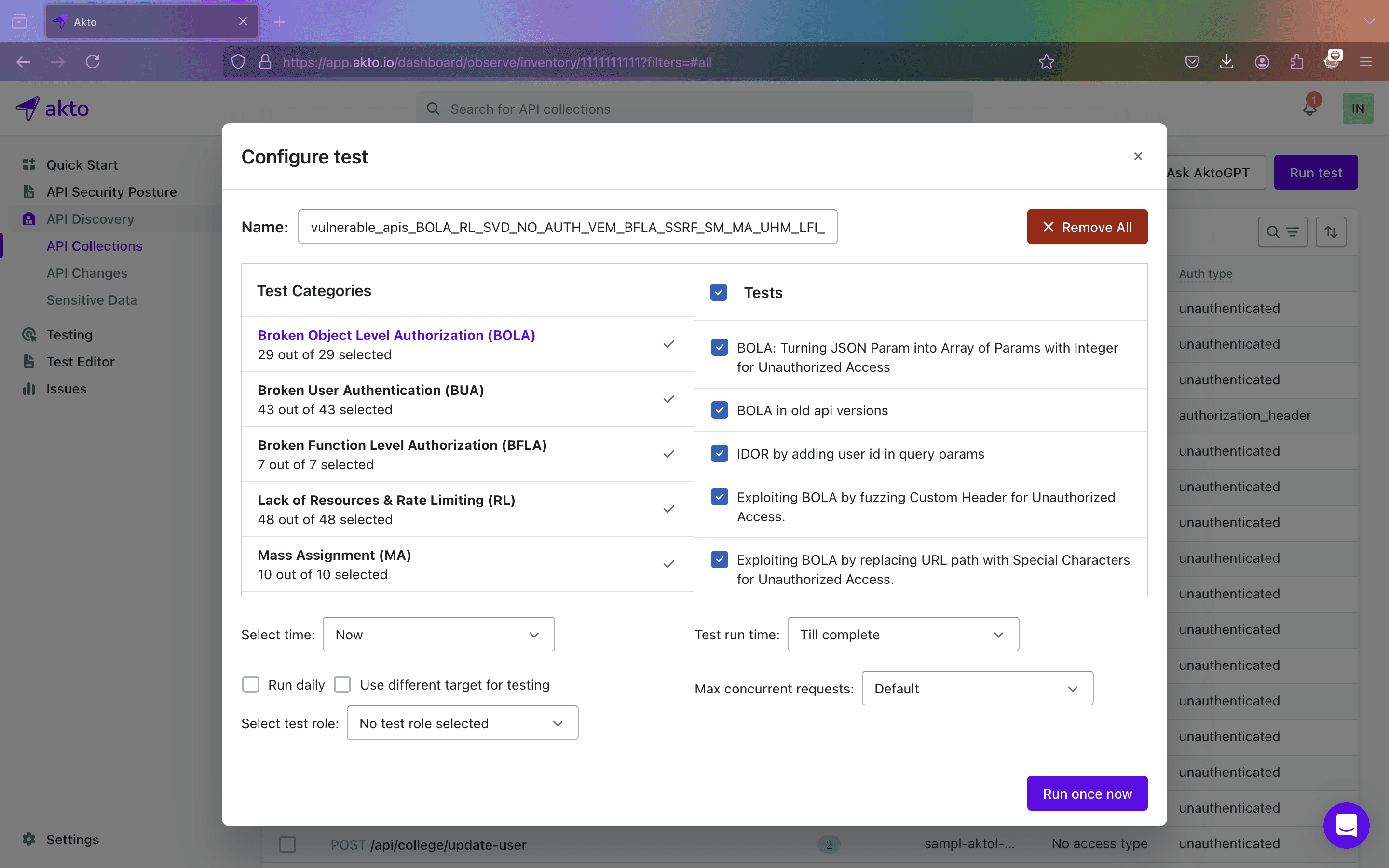
Akto covers all aspects of the latest OWASP Top 10
and HackerOne Top 10
, ensuring complete coverage. The platform distinguishes itself for its advanced test suites focusing on broken authentication and authorization. Akto maps each vulnerability discovered with associated CWE and related CVEs to provide the context of the finding to the developer.
Prevent API Security Misconfiguration
Implement these essential practices to fortify the API against security misconfigurations and protect the organization’s system from potential vulnerabilities.
Secure Default Configurations
Always change the default settings of the API to secure configurations. Attackers often publicly document and widely know default settings, such as admin credentials, open ports, or unsecured endpoints.
Security engineers must take an active role in identifying and updating these default configurations during the API setup process. By customizing these settings, engineers reduce the chances of an attacker exploiting well-known vulnerabilities. Conducting regular reviews and updates of these configurations is essential to ensure they stay aligned with the latest security best practices.
Example Change default admin passwords and disable default user accounts to prevent unauthorized access.
This code enhances API security by changing the default admin password and disabling default user accounts. In the first part, it sends a POST request to the /change-password
endpoint, updating the admin's weak default password (admin123
) to a stronger one (StrongPassword!23
). The second part sends a POST request to the /disable-account
endpoint, disabling a potentially vulnerable default user account (defaultUser
).
Configuration Reviews and Audits
Regularly review and audit the API configurations to ensure they stay secure and up to date. Security engineers should actively check for misconfigurations, outdated settings, or any unintended changes that could expose vulnerabilities.
By conducting frequent audits, security teams can catch potential security issues early before attackers can exploit them. This proactive approach helps maintain a strong security posture
and ensures that the APIs remain aligned with best practices.
Example Schedule quarterly audits to check that all security settings are up-to-date and no one has introduced insecure configurations.
In this code, a subprocess
module is used to run an external command-line tool (api-audit-tool
) that audits API configurations with the configuration file api-config.json
. The result is captured and printed to the console.
If the tool returns a non-zero exit code, it indicates a failure and a message is printed to notify the user to review the configurations. Otherwise, a success message confirms that all configurations are secure. The function is intended to be scheduled for execution quarterly to ensure API configurations remain up-to-date and secure.
Principle of Least Privilege
Always assign users and applications the minimum access necessary to perform their tasks. Security engineers should actively review and adjust permissions to ensure no one has more privileges than required.
By limiting access, security engineers reduce the attack surface and prevent unauthorized actions or data exposure. Continuously monitor access levels
and revoke any unnecessary permissions as roles and responsibilities change. Enforcing the principle of least privilege helps minimize potential damage from compromised accounts or malicious insiders.
Example
If a user only needs to read data, don't give them write or delete permissions.
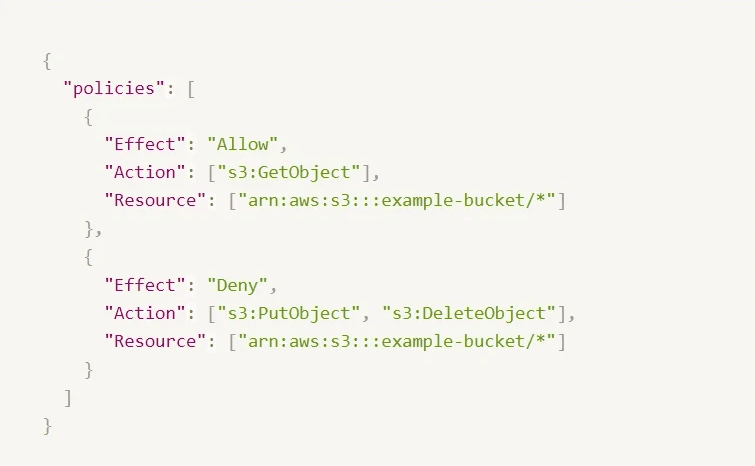
In this code, two policies control access to an Amazon S3 bucket. The first policy explicitly allows users to perform the s3:GetObject
action, granting permission to retrieve objects from the specified S3 bucket (example-bucket
).
The second policy denies users the ability to perform the s3:PutObject
and s3:DeleteObject
actions, preventing them from uploading or deleting objects in the same bucket. This setup ensures that users can only read data from the bucket but cannot modify or remove any objects, enforcing the principle of least privilege.
Proper Authentication and Authorization Mechanisms
Implement strong authentication and authorization mechanisms to secure access to the API. Security engineers must protect all API endpoints with robust authentication methods, such as OAuth
, API keys, or multi-factor authentication
(MFA).
Regularly review and update these mechanisms to keep up with evolving security standards. By enforcing proper authorization, security engineers ensure that only users with the correct permissions can access specific resources or perform sensitive actions. Continuously monitor and audit authentication and authorization processes to detect and mitigate any potential weaknesses.
Example Use techniques like OAuth 2.0 or JWT (JSON Web Tokens
) to verify users.
In this code, a Flask web application is set up with JWT-based authentication. The token_required
decorator function checks for a JWT token in the request headers under Authorization
. If the token is missing or invalid, the server returns a 403 status with an error message.
If the token is valid, it decodes the token using the SECRET_KEY
and grants access to the wrapped function. This decorator protects the /secure-data
endpoint, meaning only authorized users with a valid token can access the data.
When users access it successfully, it returns a message confirming that the resource is restricted to authorized users. The application runs when executed as the main module.
Secure API Gateway Configuration
Configure the API gateway securely to ensure it effectively manages and protects the traffic between the API and users. Security engineers should enable rate limiting to prevent abuse, such as excessive requests or brute force attacks, by limiting the number of requests an entity can make within a set time frame.
Implement TLS encryption
to protect data as it travels between users and the API, safeguarding sensitive information from interception. Use authentication mechanisms to validate requests before they reach the API, ensuring that only legitimate users can access the endpoints. Continuously monitor the gateway’s performance and logs to detect any suspicious activity and respond quickly to potential threats.
Example Set up the gateway to only allow a certain number of requests per minute from each user and use HTTPS to encrypt data.
In this Kubernetes configuration, the configuration defines a Gateway resource as a way to manage traffic for the API. The gateway listens on port 443 using the HTTPS protocol, and the certificate reference named example-cert
enables TLS encryption.
It routes any requests matching the path /api/*
to the backend service called example-service
on port 80. Additionally, the configuration enforces a rate limit, allowing a maximum of 100 requests per minute to prevent traffic abuse. This setup ensures secure and controlled access to the API via the gateway.
Final Thoughts
Properly setting up and maintaining API security configurations is crucial. Failing to do so can result in slower API performance, increased vulnerability to attacks, data breaches, unexpected downtime, and costly maintenance efforts.
By actively securing configurations, regularly reviewing them, and embedding security checks into the development process, organizations can greatly reduce these risks while keeping their APIs reliable and efficient. Akto offers comprehensive API security solutions to help organizations achieve this. Book a demo today and start safeguarding the APIs!
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.